编写程序,将输入的20名学生的成绩保存到数组中,求出其最高分、最低分及平均分。 编写程序,将输入的20名学生的成绩保存到数组中,求出其最高分、最低分及平均分。 输入格式: 11 12 23 36 48 56 72 78 89 96 78 89 90 100 78 89 56 67 45 98 输出格式: max=100.0 min=11.0 avg=65.6
时间: 2024-01-24 22:19:03 浏览: 110
以下是Python代码实现:
```python
scores = input().split() # 输入成绩,以空格分隔,并转换为字符串列表
scores = list(map(float, scores)) # 将字符串列表转换为浮点数列表
max_score = max(scores) # 求最高分
min_score = min(scores) # 求最低分
avg_score = sum(scores) / len(scores) # 求平均分
print("max={:.1f} min={:.1f} avg={:.1f}".format(max_score, min_score, avg_score)) # 输出结果
```
使用`input()`函数获取输入,将输入的字符串以空格分割并转换为浮点数列表。使用Python内置的`max`和`min`函数求得最高分和最低分,使用`sum`函数求和并除以列表长度求得平均分。最后使用`print`函数输出结果,使用字符串格式化将结果格式化为指定格式输出。
相关问题
编写程序,将输入的20名学生的成绩保存到数组中,求出其最高分,最低分及平均分
编写这样的程序,通常需要以下步骤:
1. **创建数组**:首先定义一个可以存储20个学生成绩的数组,比如`double[] scores = new double[20];`
2. **获取输入**:使用循环结构如for循环,提示用户依次输入每个学生的成绩,并将其存储到数组中。
```java
for (int i = 0; i < 20; i++) {
System.out.println("请输入第 " + (i+1) + " 名学生的成绩:");
scores[i] =.nextDouble(); // 假设你是Java环境,这里使用Scanner的nextDouble()方法
}
```
3. **查找最高分和最低分**:遍历数组,分别记录下当前找到的最大值(最高分)和最小值(最低分)。
```java
double maxScore = scores[0];
double minScore = scores[0];
for (double score : scores) {
if (score > maxScore) {
maxScore = score;
}
if (score < minScore) {
minScore = score;
}
}
```
4. **计算平均分**:遍历完整个数组后,可以用总分数除以学生人数得到平均分。
```java
double sum = 0;
for (double score : scores) {
sum += score;
}
double averageScore = sum / scores.length;
```
5. **输出结果**:最后,打印出最高分、最低分和平均分。
```java
System.out.println("最高分:" + maxScore);
System.out.println("最低分:" + minScore);
System.out.println("平均分:" + averageScore);
```
完整的Java代码示例:
```java
import java.util.Scanner;
public class GradeCalculator {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
double[] scores = new double[20];
for (int i = 0; i < 20; i++) {
System.out.println("请输入第 " + (i+1) + " 名学生的成绩:");
scores[i] = input.nextDouble();
}
double maxScore = scores[0];
double minScore = scores[0];
double sum = 0;
for (double score : scores) {
if (score > maxScore) {
maxScore = score;
} else if (score < minScore) {
minScore = score;
}
sum += score;
}
double averageScore = sum / scores.length;
System.out.println("最高分:" + maxScore);
System.out.println("最低分:" + minScore);
System.out.println("平均分:" + averageScore);
}
}
```
编写程序,将输入的20名学生的成绩保存到数组中,求出其最高分、最低分及平均分C语言代码
#include <stdio.h>
int main() {
int scores[20];
int sum = 0;
int max = 0;
int min = 100;
// 输入成绩
for (int i = 0; i < 20; i++) {
printf("请输入第%d个学生的成绩:", i+1);
scanf("%d", &scores[i]);
// 求和
sum += scores[i];
// 求最高分
if (scores[i] > max) {
max = scores[i];
}
// 求最低分
if (scores[i] < min) {
min = scores[i];
}
}
// 求平均分
float average = (float)sum / 20.0;
// 输出结果
printf("最高分:%d\n", max);
printf("最低分:%d\n", min);
printf("平均分:%f\n", average);
return 0;
}
阅读全文
相关推荐
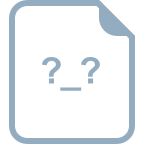













