用c语言写:完成增加商品,按照学号删除商品,修改商品信息,查询商品信息的
时间: 2024-03-12 09:49:21 浏览: 130
代码如下:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 50
#define MAX_PRODUCTS 20
typedef struct {
char id[MAX_LEN];
char name[MAX_LEN];
double price;
} Product;
int find_product_index(Product products[], int num_products, char id[]) {
for (int i = 0; i < num_products; i++) {
if (strcmp(products[i].id, id) == 0) {
return i;
}
}
return -1;
}
void add_product(Product products[], int *num_products) {
if (*num_products == MAX_PRODUCTS) {
printf("Error: Maximum number of products reached!\n");
return;
}
Product new_product;
printf("Enter product ID: ");
scanf("%s", new_product.id);
printf("Enter product name: ");
scanf("%s", new_product.name);
printf("Enter product price: ");
scanf("%lf", &new_product.price);
products[*num_products] = new_product;
(*num_products)++;
printf("Product added successfully!\n");
}
void delete_product(Product products[], int *num_products) {
char id[MAX_LEN];
printf("Enter product ID to delete: ");
scanf("%s", id);
int index = find_product_index(products, *num_products, id);
if (index == -1) {
printf("Error: Product not found!\n");
return;
}
for (int i = index; i < (*num_products) - 1; i++) {
products[i] = products[i + 1];
}
(*num_products)--;
printf("Product deleted successfully!\n");
}
void modify_product(Product products[], int num_products) {
char id[MAX_LEN];
printf("Enter product ID to modify: ");
scanf("%s", id);
int index = find_product_index(products, num_products, id);
if (index == -1) {
printf("Error: Product not found!\n");
return;
}
Product modified_product;
printf("Enter new product name (press enter to skip): ");
scanf("%s", modified_product.name);
printf("Enter new product price (press 0 to skip): ");
scanf("%lf", &modified_product.price);
if (strlen(modified_product.name) > 0) {
strcpy(products[index].name, modified_product.name);
}
if (modified_product.price != 0) {
products[index].price = modified_product.price;
}
printf("Product modified successfully!\n");
}
void display_product(Product product) {
printf("%-10s %-20s $%.2lf\n", product.id, product.name, product.price);
}
void display_all_products(Product products[], int num_products) {
printf("%-10s %-20s %s\n", "ID", "Name", "Price");
for (int i = 0; i < num_products; i++) {
display_product(products[i]);
}
}
int main() {
Product products[MAX_PRODUCTS];
int num_products = 0;
int choice;
do {
printf("\n1. Add product\n");
printf("2. Delete product\n");
printf("3. Modify product\n");
printf("4. Display all products\n");
printf("0. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_product(products, &num_products);
break;
case 2:
delete_product(products, &num_products);
break;
case 3:
modify_product(products, num_products);
break;
case 4:
display_all_products(products, num_products);
break;
case 0:
printf("Exiting program...\n");
break;
default:
printf("Invalid choice!\n");
}
} while (choice != 0);
return 0;
}
```
这个程序定义了一个 `Product` 结构体,包含商品的 ID、名称和价格。然后实现了增加商品、按学号删除商品、修改商品信息和查询商品信息的功能。其中,查询商品信息的功能是通过 `display_all_products` 函数实现的,该函数将所有商品的信息输出到控制台上。
阅读全文
相关推荐
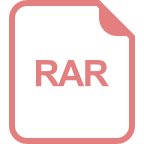
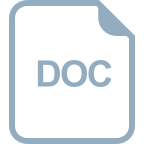
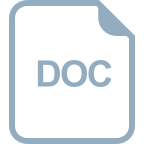
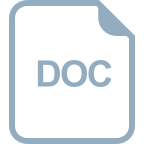



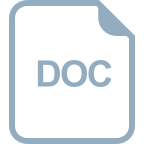
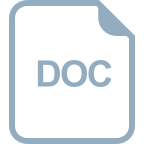
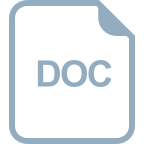
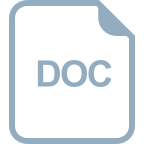
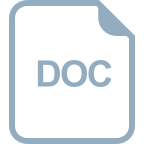
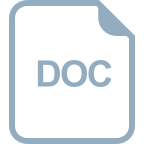
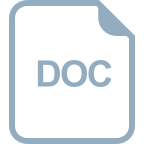


