JAVA模拟三个老师同时给十个小朋友发礼品,每个老师相当于一个线程
时间: 2023-07-20 10:33:47 浏览: 53
好的,这是一个典型的生产者-消费者模型问题。我们可以使用 Java 中的多线程技术来实现。具体步骤如下:
1. 定义一个礼品类 Gift,包含礼品名称和礼品数量属性。
```java
public class Gift {
private String name;
private int count;
public Gift(String name, int count) {
this.name = name;
this.count = count;
}
public String getName() {
return name;
}
public int getCount() {
return count;
}
public void setCount(int count) {
this.count = count;
}
}
```
2. 定义一个礼品列表类 GiftList,包含一个 Gift 数组和一个计数器,用于统计礼品总数。
```java
public class GiftList {
private Gift[] gifts;
private int count;
public GiftList(int size) {
gifts = new Gift[size];
count = 0;
}
public synchronized boolean addGift(Gift gift) {
if (count >= gifts.length) {
return false;
}
gifts[count] = gift;
count++;
return true;
}
public int getCount() {
return count;
}
}
```
3. 定义一个生产者类 Teacher,代表一个老师。每个老师都有自己的礼品列表和礼品数量,可以向礼品列表中添加礼品。
```java
public class Teacher implements Runnable {
private String name;
private GiftList giftList;
private int giftCount;
public Teacher(String name, GiftList giftList, int giftCount) {
this.name = name;
this.giftList = giftList;
this.giftCount = giftCount;
}
public void run() {
for (int i = 0; i < giftCount; i++) {
Gift gift = new Gift(name + "的礼品" + (i + 1), 1);
while (!giftList.addGift(gift)) {
Thread.yield();
}
System.out.println(name + "发放了礼品" + (i + 1));
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
4. 定义一个消费者类 Child,代表一个小朋友。每个小朋友都从礼品列表中取出一件礼品,并将礼品数量减 1。
```java
public class Child implements Runnable {
private String name;
private GiftList giftList;
public Child(String name, GiftList giftList) {
this.name = name;
this.giftList = giftList;
}
public void run() {
while (giftList.getCount() > 0) {
Gift gift = null;
synchronized (giftList) {
if (giftList.getCount() > 0) {
gift = giftList.getGift();
}
}
if (gift != null) {
System.out.println(name + "收到了礼品:" + gift.getName());
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
```
5. 在主函数中创建三个 Teacher 实例和十个 Child 实例,并分别启动它们的线程。
```java
public static void main(String[] args) {
GiftList giftList = new GiftList(30);
Thread[] teacherThreads = new Thread[3];
for (int i = 0; i < teacherThreads.length; i++) {
Teacher teacher = new Teacher("老师" + (i + 1), giftList, 10);
teacherThreads[i] = new Thread(teacher);
teacherThreads[i].start();
}
Thread[] childThreads = new Thread[10];
for (int i = 0; i < childThreads.length; i++) {
Child child = new Child("小朋友" + (i + 1), giftList);
childThreads[i] = new Thread(child);
childThreads[i].start();
}
}
```
这样就可以模拟三个老师同时给十个小朋友发礼品的场景了。
相关推荐
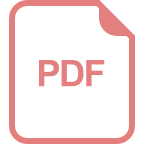














