用HTML,CSS,js,php,sql实现评论回复页面功能
时间: 2024-03-08 21:47:39 浏览: 198
以下是一个简单的评论回复页面的实现方式,使用 HTML、CSS、JavaScript、PHP 和 SQL:
HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>评论回复</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>评论回复</h1>
<!-- 评论表单 -->
<form method="post" action="post_comment.php">
<h2>发表评论</h2>
<input type="text" name="name" placeholder="姓名" required>
<input type="email" name="email" placeholder="邮箱" required>
<textarea name="comment" placeholder="输入评论" required></textarea>
<input type="submit" value="提交评论">
</form>
<!-- 评论列表 -->
<h2>评论列表</h2>
<div id="comments">
<!-- 评论数据通过 PHP 从数据库中读取并显示 -->
<?php include "get_comments.php"; ?>
</div>
<!-- 回复表单 -->
<div id="reply-form">
<h2>回复评论</h2>
<form method="post" action="post_reply.php">
<input type="hidden" name="comment_id" id="comment_id">
<input type="text" name="name" placeholder="姓名" required>
<input type="email" name="email" placeholder="邮箱" required>
<textarea name="comment" placeholder="输入回复" required></textarea>
<input type="submit" value="提交回复">
<button type="button" onclick="hideReplyForm()">取消</button>
</form>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS 代码:
```css
body {
font-family: Arial, sans-serif;
}
h1 {
text-align: center;
margin-top: 20px;
}
h2 {
margin-top: 30px;
margin-bottom: 10px;
}
form {
margin-bottom: 20px;
padding: 20px;
background-color: #f5f5f5;
border-radius: 5px;
}
input[type=text], input[type=email], textarea {
display: block;
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: none;
border-radius: 5px;
background-color: #fff;
box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
}
input[type=submit], button {
display: inline-block;
padding: 10px 20px;
border: none;
border-radius: 5px;
background-color: #007bff;
color: #fff;
cursor: pointer;
}
button {
margin-left: 10px;
background-color: #dc3545;
}
#comments {
background-color: #f5f5f5;
border-radius: 5px;
padding: 20px;
}
.comment {
margin-bottom: 20px;
padding: 10px;
border: 1px solid #ccc;
background-color: #fff;
border-radius: 5px;
}
.comment h3 {
margin-top: 0;
}
.comment p {
margin-bottom: 0;
}
.comment .reply {
margin-top: 10px;
margin-left: 20px;
font-style: italic;
}
```
JavaScript 代码:
```javascript
// 显示回复表单
function showReplyForm(commentId) {
document.getElementById("comment_id").value = commentId;
document.getElementById("reply-form").style.display = "block";
}
// 隐藏回复表单
function hideReplyForm() {
document.getElementById("reply-form").style.display = "none";
}
```
PHP 代码(get_comments.php):
```php
<?php
// 连接数据库
$conn = mysqli_connect("localhost", "username", "password", "database_name");
// 查询评论列表
$sql = "SELECT * FROM comments ORDER BY created_at DESC";
$result = mysqli_query($conn, $sql);
// 遍历评论列表并输出 HTML 代码
while ($row = mysqli_fetch_assoc($result)) {
echo "<div class='comment'>";
echo "<h3>{$row['name']}:</h3>";
echo "<p>{$row['comment']}</p>";
echo "<button onclick='showReplyForm({$row['id']})'>回复</button>";
// 查询回复列表
$sql2 = "SELECT * FROM replies WHERE comment_id={$row['id']}";
$result2 = mysqli_query($conn, $sql2);
// 遍历回复列表并输出 HTML 代码
while ($row2 = mysqli_fetch_assoc($result2)) {
echo "<div class='reply'>";
echo "<h4>{$row2['name']}:</h4>";
echo "<p>{$row2['comment']}</p>";
echo "</div>";
}
echo "</div>";
}
// 关闭数据库连接
mysqli_close($conn);
?>
```
PHP 代码(post_comment.php):
```php
<?php
// 获取表单数据
$name = $_POST["name"];
$email = $_POST["email"];
$comment = $_POST["comment"];
// 连接数据库
$conn = mysqli_connect("localhost", "username", "password", "database_name");
// 插入评论数据
$sql = "INSERT INTO comments (name, email, comment) VALUES ('$name', '$email', '$comment')";
mysqli_query($conn, $sql);
// 关闭数据库连接
mysqli_close($conn);
// 返回评论页面
header("Location: index.php");
?>
```
PHP 代码(post_reply.php):
```php
<?php
// 获取表单数据
$commentId = $_POST["comment_id"];
$name = $_POST["name"];
$email = $_POST["email"];
$comment = $_POST["comment"];
// 连接数据库
$conn = mysqli_connect("localhost", "username", "password", "database_name");
// 插入回复数据
$sql = "INSERT INTO replies (comment_id, name, email, comment) VALUES ($commentId, '$name', '$email', '$comment')";
mysqli_query($conn, $sql);
// 关闭数据库连接
mysqli_close($conn);
// 返回评论页面
header("Location: index.php");
?>
```
SQL 代码:
```sql
CREATE TABLE comments (
id INT(11) NOT NULL AUTO_INCREMENT,
name VARCHAR(50) NOT NULL,
email VARCHAR(50) NOT NULL,
comment TEXT NOT NULL,
created_at DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
updated_at DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (id)
);
CREATE TABLE replies (
id INT(11) NOT NULL AUTO_INCREMENT,
comment_id INT(11) NOT NULL,
name VARCHAR(50) NOT NULL,
email VARCHAR(50) NOT NULL,
comment TEXT NOT NULL,
created_at DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP,
updated_at DATETIME NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (id)
);
ALTER TABLE replies ADD CONSTRAINT fk_comment_id FOREIGN KEY (comment_id) REFERENCES comments(id);
```
以上是一个简单的评论回复页面的实现方式,可以根据实际需求进行修改和扩展。
阅读全文
相关推荐




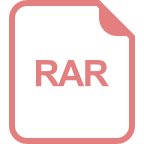





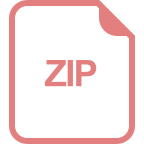


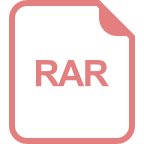




