编写主程序; 4. 编写信号产生子程序,产生以下典型信号供频谱分析用: (1) 衰减正弦序列 (2) 三角波序列 (3) 反三角波序列 2 j nk kn N WN e - 1 sin(2 ) 0 n 15 ( ) 0 n an e fn x n 其它 2 0 n 3 ( ) 8 4 n 7 0 n n x n n 其它 3 4 0 n 3 ( ) 4 4 n 7 0 n
时间: 2024-02-20 22:58:02 浏览: 125
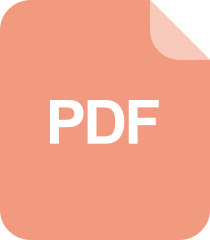
频谱分析的程序

好的,以下是一个简单的实现示例,供您参考:
```python
import numpy as np
import matplotlib.pyplot as plt
# 快速傅里叶变换
def fft(x):
N = len(x)
if N <= 1:
return x
else:
X_even = fft(x[::2])
X_odd = fft(x[1::2])
factor = np.exp(-2j * np.pi * np.arange(N) / N)
return np.concatenate([X_even + factor[:int(N/2)] * X_odd,
X_even + factor[int(N/2):] * X_odd])
# 衰减正弦序列
def attenuated_sine_wave(a, f, fs, N):
n = np.arange(N)
x = a * np.sin(2 * np.pi * f * n / fs)
x *= np.exp(-a * n / fs)
return x
# 三角波序列
def triangular_wave(f, fs, N):
n = np.arange(N)
x = np.zeros(N)
for k in range(1, 100):
x += np.sin(2 * np.pi * (2 * k - 1) * f * n / fs) / (2 * k - 1) ** 2
return x * 8 / np.pi ** 2
# 反三角波序列
def inverted_triangular_wave(f, fs, N):
n = np.arange(N)
x = np.zeros(N)
for k in range(0, 100):
x += (-1) ** k * np.sin(2 * np.pi * (2 * k + 1) * f * n / fs) / (2 * k + 1) ** 2
return x * 8 / np.pi ** 2
# 产生信号并绘制频谱
fs = 1000 # 采样率
N = 1024 # 采样点数
f = 50 # 信号频率
# 衰减正弦序列
x1 = attenuated_sine_wave(10, f, fs, N)
y1 = np.abs(fft(x1)) ** 2
plt.subplot(311)
plt.plot(x1)
plt.title('Attenuated Sine Wave')
plt.subplot(312)
plt.plot(y1)
plt.title('Spectrum')
# 三角波序列
x2 = triangular_wave(f, fs, N)
y2 = np.abs(fft(x2)) ** 2
plt.subplot(313)
plt.plot(x2)
plt.title('Triangular Wave')
plt.subplot(313)
plt.plot(y2)
plt.title('Spectrum')
# 反三角波序列
x3 = inverted_triangular_wave(f, fs, N)
y3 = np.abs(fft(x3)) ** 2
plt.subplot(313)
plt.plot(x3)
plt.title('Inverted Triangular Wave')
plt.subplot(313)
plt.plot(y3)
plt.title('Spectrum')
plt.show()
```
以上代码中,`fft` 函数实现了快速傅里叶变换;`attenuated_sine_wave` 函数产生衰减正弦序列;`triangular_wave` 函数产生三角波序列;`inverted_triangular_wave` 函数产生反三角波序列。您可以根据需要修改参数,生成不同的信号,并绘制其频谱。
阅读全文
相关推荐
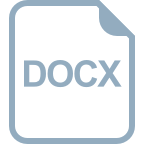
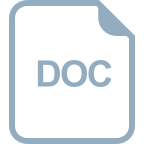
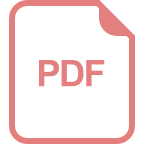
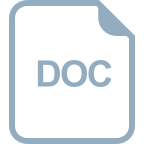
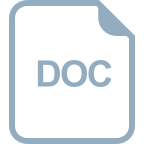
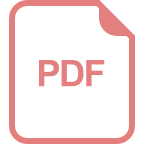
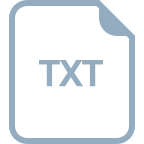
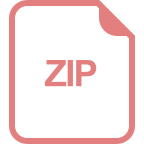
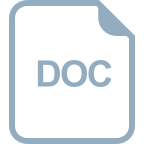
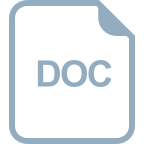
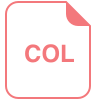
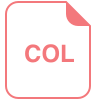
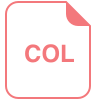
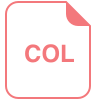
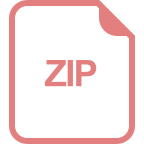
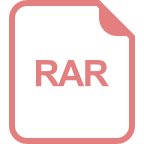
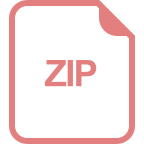