java里的IOUtil.write
时间: 2023-05-29 13:02:15 浏览: 298
IOUtil.write是一个静态方法,用于将数据写入输出流中。
它的方法签名为:public static int write(InputStream in, OutputStream out, int bufferSize) throws IOException。
其中,参数in是需要写入的输入流,参数out是需要将数据写入的输出流,参数bufferSize是缓冲区的大小。
该方法返回成功写入的字节数。
以下是一个示例使用IOUtil.write方法将一个输入流写入到一个输出流中:
InputStream in = new FileInputStream("input.txt");
OutputStream out = new FileOutputStream("output.txt");
IOUtil.write(in, out, 1024);
in.close();
out.close();
相关问题
ioutil.TempFile替代
如果你想替代使用`ioutil.TempFile`,你可以尝试使用`os.CreateTemp`函数。下面是一个示例代码:
```go
package main
import (
"fmt"
"io/ioutil"
"os"
)
func main() {
// 创建临时文件
tempFile, err := ioutil.TempFile("", "example")
if err != nil {
fmt.Println("创建临时文件失败:", err)
return
}
defer os.Remove(tempFile.Name()) // 确保程序退出时删除临时文件
fmt.Println("临时文件名:", tempFile.Name())
// 使用临时文件进行操作
_, err = tempFile.WriteString("Hello, World!")
if err != nil {
fmt.Println("写入临时文件失败:", err)
return
}
// 读取临时文件
data, err := ioutil.ReadFile(tempFile.Name())
if err != nil {
fmt.Println("读取临时文件失败:", err)
return
}
fmt.Println("临时文件内容:", string(data))
}
```
在这个示例中,我们使用`ioutil.TempFile`创建一个临时文件,并使用`os.Remove`在程序退出时删除它。然后我们可以像操作普通文件一样对临时文件进行读写操作。
Java里的socketChannel.write
Java中的SocketChannel是一种用于非阻塞I/O的通道,可以进行网络连接操作,同时还可以通过该通道读写数据。
SocketChannel.write()方法是用来将数据从Buffer写入到SocketChannel中的。它具有以下语法:
public abstract int write(ByteBuffer src) throws IOException
其中,src为待写入数据的ByteBuffer对象。该方法的返回值表示实际写入的字节数,如果返回0表示目前无法写入数据;如果返回-1表示连接已经关闭。
使用 SocketChannel.write() 方法需要遵循以下步骤:
1. 创建SocketChannel对象,并打开连接。
2. 创建ByteBuffer对象,将待写入数据写入该对象。
3. 使用SocketChannel.write()方法将ByteBuffer中的数据写入到SocketChannel中。
4. 检查SocketChannel.write()方法的返回值(即写入的结果)。
5. 若写入结果为0,说明目前无法进行写入操作,需要等待一段时间后再次尝试。
6. 若写入结果为-1,说明连接已经关闭,需要关闭相关资源并退出程序。
7. 若写入结果为正整数,则表示成功写入了对应的字节数。
相关推荐
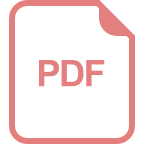
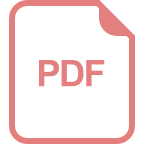
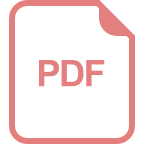












