#include<stdio.h> struct people{ char id[8]; char age; int name; } main() { struct people peo[3],*p; p=peo; (*p).id[8]="lihua"; (*p).name=18; (*++p).id[8]="huahua"; (*++p).name=20; (*++p).id[8]="lixiao"; (*++p).name=24; for(int i=0;i<3;i++) { printf("%s,%d",(*p).id,(*p).name ); p++; } }
时间: 2023-12-10 09:42:01 浏览: 93
这段代码有一些问题,可能会导致程序崩溃或输出错误的结果。我来帮你解释一下。
首先,结构体 `people` 中的 `name` 字段的类型是 `int`,但在代码中却用了一个 `char` 类型的变量进行赋值。这会导致数据类型不匹配的问题。建议将 `age` 改为 `int` 类型。
其次,结构体数组 `peo` 的大小为 3,但在代码中却对其进行了 4 次赋值。这会导致数组越界访问的问题。建议将数组大小改为 4。
最后,字符串的赋值应该使用 strcpy() 函数,而不是直接赋值。另外,结构体成员的访问应该使用箭头运算符 ->,而不是 (*p).id 的形式。
下面是修改后的代码:
```
#include <stdio.h>
#include <string.h>
struct people {
char id[8];
int age;
int name;
};
int main() {
struct people peo[4], *p;
p = peo;
strcpy((*p).id, "lihua");
(*p).name = 18;
strcpy((*++p).id, "huahua");
(*p).name = 20;
strcpy((*++p).id, "lixiao");
(*p).name = 24;
for (int i = 0; i < 3; i++) {
printf("%s,%d\n", p->id, p->name);
p++;
}
return 0;
}
```
阅读全文
相关推荐



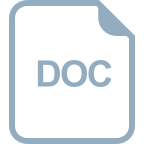

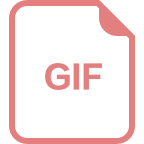










