帮我写一个STM32 自定义HID通信的示例程序
时间: 2023-12-07 20:04:35 浏览: 245
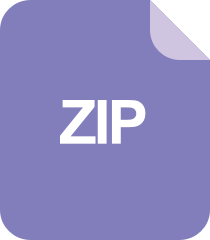
STM32 自定义HID 设备, 通过快传输接收上位机发来的数据。上位机使用Libusb库函数实现,接口简单

好的,以下是一个基于STM32的自定义HID通信示例程序,使用HAL库进行驱动,代码注释中有详细的说明:
```c
#include "main.h"
#include "usbd_core.h"
#include "usbd_desc.h"
#include "usbd_hid.h"
#include "usbd_hid_if.h"
// USB Device Handle
USBD_HandleTypeDef hUsbDeviceFS;
// HID Report Descriptor
__ALIGN_BEGIN static uint8_t HID_ReportDesc_FS[USBD_HID_REPORT_DESC_SIZE] __ALIGN_END = {
0x06, 0x00, 0xFF, // USAGE_PAGE (Vendor Defined Page 1)
0x09, 0x01, // USAGE (Vendor Usage 1)
0xA1, 0x01, // COLLECTION (Application)
0x15, 0x00, // LOGICAL_MINIMUM (0)
0x26, 0xFF, 0x00, // LOGICAL_MAXIMUM (255)
0x75, 0x08, // REPORT_SIZE (8)
0x95, 0x40, // REPORT_COUNT (64)
0x09, 0x01, // USAGE (Vendor Usage 1)
0x81, 0x02, // INPUT (Data,Var,Abs)
0x09, 0x01, // USAGE (Vendor Usage 1)
0x91, 0x02, // OUTPUT (Data,Var,Abs)
0xC0 // END_COLLECTION
};
// USB Device Descriptor
__ALIGN_BEGIN static uint8_t USBD_DeviceDesc[USB_LEN_DEV_DESC] __ALIGN_END = {
0x12, // bLength
USB_DESC_TYPE_DEVICE, // bDescriptorType
0x00, // bcdUSB
0x02, // bcdUSB
0xFF, // bDeviceClass
0x00, // bDeviceSubClass
0x00, // bDeviceProtocol
USB_MAX_EP0_SIZE, // bMaxPacketSize
LOBYTE(USB_VID), // idVendor
HIBYTE(USB_VID), // idVendor
LOBYTE(USB_PID), // idProduct
HIBYTE(USB_PID), // idProduct
0x00, // bcdDevice rel. 2.00
0x02, // bcdDevice rel. 2.00
USBD_IDX_MFC_STR, // Index of manufacturer string
USBD_IDX_PRODUCT_STR, // Index of product string
USBD_IDX_SERIAL_STR, // Index of serial number string
USBD_MAX_NUM_CONFIGURATION // bNumConfigurations
};
// USB HID Configuration Descriptor
__ALIGN_BEGIN static uint8_t USBD_HID_Desc[USB_HID_DESC_SIZ] __ALIGN_END = {
0x09, // bLength: HID Descriptor size
HID_DESCRIPTOR_TYPE, // bDescriptorType: HID
0x11, 0x01, // bcdHID: HID Class Spec release number
0x00, // bCountryCode: Hardware target country
0x01, // bNumDescriptors: Number of HID class descriptors to follow
0x22, // bDescriptorType
sizeof(HID_ReportDesc_FS), // wItemLength: Total length of Report descriptor
0x00
};
// USB HID Configuration
__ALIGN_BEGIN static uint8_t USBD_HID_CfgDesc[USB_HID_CONFIG_DESC_SIZ] __ALIGN_END = {
0x09, // bLength: Configuration Descriptor size
USB_DESC_TYPE_CONFIGURATION, // bDescriptorType: Configuration
USB_HID_CONFIG_DESC_SIZ, 0x00, // wTotalLength: Total length of the configuration
0x01, // bNumInterfaces: Number of interfaces
0x01, // bConfigurationValue: Configuration value
0x00, // iConfiguration: Index of string descriptor describing the configuration
0xC0, // bmAttributes: Bus powered and no remote wakeup
0x32, // MaxPower 100 mA: this current is used for detecting Vbus
// Interface Descriptor
0x09, // bLength: Interface Descriptor size
USB_DESC_TYPE_INTERFACE, // bDescriptorType: Interface
0x00, // bInterfaceNumber: Number of Interface
0x00, // bAlternateSetting: Alternate setting
0x02, // bNumEndpoints
0x03, // bInterfaceClass: HID
0x00, // bInterfaceSubClass: 1=BOOT, 0=no boot
0x00, // bInterfaceProtocol: 0=none, 1=keyboard, 2=mouse
0x00, // iInterface: Index of string descriptor
// HID Descriptor
0x09, // bLength: HID Descriptor size
HID_DESCRIPTOR_TYPE, // bDescriptorType: HID
0x11, 0x01, // bcdHID: HID Class Spec release number
0x00, // bCountryCode: Hardware target country
0x01, // bNumDescriptors: Number of HID class descriptors to follow
0x22, // bDescriptorType
sizeof(HID_ReportDesc_FS), // wItemLength: Total length of Report descriptor
0x00,
// Endpoint Descriptor
0x07, // bLength: Endpoint Descriptor size
USB_DESC_TYPE_ENDPOINT, // bDescriptorType: Endpoint
HID_EPIN_ADDR, // bEndpointAddress: Endpoint Address (IN)
USBD_EP_TYPE_INTR, // bmAttributes: Interrupt endpoint
LOBYTE(HID_EPIN_SIZE), // wMaxPacketSize: 64 bytes max
HIBYTE(HID_EPIN_SIZE),
HID_FS_BINTERVAL, // bInterval: Polling Interval (10 ms)
// Endpoint Descriptor
0x07, // bLength: Endpoint Descriptor size
USB_DESC_TYPE_ENDPOINT, // bDescriptorType: Endpoint
HID_EPOUT_ADDR, // bEndpointAddress: Endpoint Address (OUT)
USBD_EP_TYPE_INTR, // bmAttributes: Interrupt endpoint
LOBYTE(HID_EPOUT_SIZE), // wMaxPacketSize: 64 bytes max
HIBYTE(HID_EPOUT_SIZE),
HID_FS_BINTERVAL // bInterval: Polling Interval (10 ms)
};
// USB Device Initialization Function
static void MX_USB_DEVICE_Init(void)
{
// Init Device Library
USBD_Init(&hUsbDeviceFS, &FS_Desc, DEVICE_FS);
// Add Supported Class
USBD_RegisterClass(&hUsbDeviceFS, &USBD_HID);
// Add HID Interface
USBD_HID_RegisterInterface(&hUsbDeviceFS, &USBD_HID_fops);
// Start Device Process
USBD_Start(&hUsbDeviceFS);
}
int main(void)
{
// Initialize HAL Library
HAL_Init();
// Configure the System Clock
SystemClock_Config();
// Initialize USB Device
MX_USB_DEVICE_Init();
while (1)
{
// Main Loop
}
}
void HAL_GPIO_EXTI_Callback(uint16_t GPIO_Pin)
{
// Handle EXTI Interrupts
if (GPIO_Pin == GPIO_PIN_13)
{
// Send HID Report
uint8_t data[64] = {0}; // HID Report Data
data[0] = 0x01; // Report ID
data[1] = HAL_GPIO_ReadPin(GPIOC, GPIO_PIN_13); // Button State
USBD_HID_SendReport(&hUsbDeviceFS, data, 64);
}
}
// USB Interrupt Handler
void OTG_FS_IRQHandler(void)
{
HAL_PCD_IRQHandler(&hUsbDeviceFS.pcd);
}
```
使用该示例程序时,需要先在CubeMX中配置USB Device和HID功能,然后生成代码并添加相应的驱动文件,具体操作可以参考ST官方文档。在本示例程序中,我们通过PC13引脚的状态来控制HID Report的发送,当PC13引脚被按下时,就会发送一个包含Report ID和Button State的HID Report。你也可以根据自己的需求来修改此处的代码。注意,该示例程序只是一个简单的示例,实际应用中需要根据自己的需求来编写相应的代码。
阅读全文
相关推荐
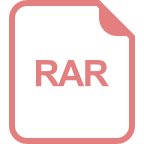
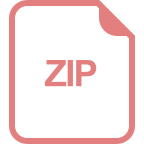
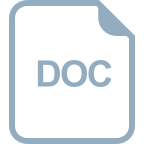
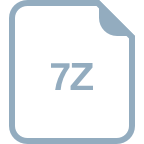
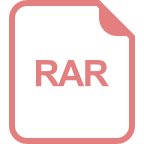
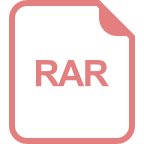
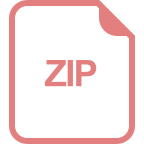
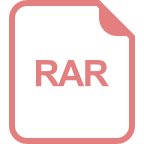
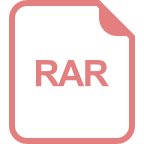
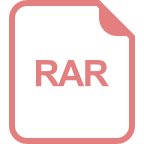
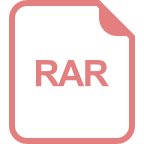
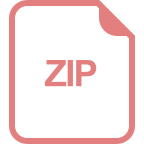
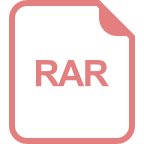
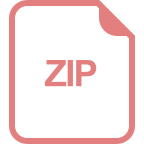
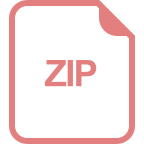
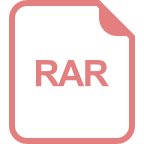