帮我用C++写一份完整的图书管理系统,复杂一点,能在控制台进行操作,需要详细的代码注释和需要设计基类和派生类,需要书籍类、图书类型类、图书类,要有较为完整的代码注释。
时间: 2024-03-04 18:51:19 浏览: 18
好的,下面是一份C++的图书管理系统示例代码,包括了基类和派生类的设计、增删改查等功能,并在控制台进行操作。代码中也包含了注释,以方便您理解。请注意,这份代码可能并不是最完美的,您可以根据自己的需求进行修改和完善。
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 基类:图书类型
class BookType {
private:
int typeId; // 图书类型编号
string typeName; // 图书类型名称
public:
BookType(int id, string name) {
typeId = id;
typeName = name;
}
int getTypeId() {
return typeId;
}
string getTypeName() {
return typeName;
}
};
// 基类:图书
class Book {
private:
int id; // 图书编号
string name; // 图书名称
string author; // 作者
int typeId; // 图书类型编号
string publish; // 出版社
int price; // 价格
public:
Book(int id, string name, string author, int typeId, string publish, int price) {
this->id = id;
this->name = name;
this->author = author;
this->typeId = typeId;
this->publish = publish;
this->price = price;
}
int getId() {
return id;
}
string getName() {
return name;
}
string getAuthor() {
return author;
}
int getTypeId() {
return typeId;
}
string getPublish() {
return publish;
}
int getPrice() {
return price;
}
};
// 派生类:书籍
class BookItem : public Book {
private:
int quantity; // 数量
public:
BookItem(int id, string name, string author, int typeId, string publish, int price, int quantity) : Book(id, name, author, typeId, publish, price) {
this->quantity = quantity;
}
int getQuantity() {
return quantity;
}
void setQuantity(int quantity) {
this->quantity = quantity;
}
};
// 图书管理系统
class BookManager {
private:
vector<BookType> bookTypes; // 图书类型列表
vector<BookItem> books; // 图书列表
public:
// 添加图书类型
void addBookType(BookType bookType) {
bookTypes.push_back(bookType);
cout << "添加图书类型成功!" << endl;
}
// 删除图书类型
void removeBookType(int typeId) {
for (vector<BookType>::iterator it = bookTypes.begin(); it != bookTypes.end();) {
if ((*it).getTypeId() == typeId) {
it = bookTypes.erase(it);
cout << "删除图书类型成功!" << endl;
}
else {
it++;
}
}
}
// 添加图书
void addBook(BookItem book) {
books.push_back(book);
cout << "添加图书成功!" << endl;
}
// 删除图书
void removeBook(int bookId) {
for (vector<BookItem>::iterator it = books.begin(); it != books.end();) {
if ((*it).getId() == bookId) {
it = books.erase(it);
cout << "删除图书成功!" << endl;
}
else {
it++;
}
}
}
// 修改图书
void updateBook(int bookId, int quantity) {
for (vector<BookItem>::iterator it = books.begin(); it != books.end(); it++) {
if ((*it).getId() == bookId) {
(*it).setQuantity(quantity);
cout << "修改图书成功!" << endl;
return;
}
}
cout << "未找到对应图书!" << endl;
}
// 查询图书
void searchBook(int typeId) {
for (vector<BookItem>::iterator it = books.begin(); it != books.end(); it++) {
if ((*it).getTypeId() == typeId) {
cout << "编号:" << (*it).getId() << endl;
cout << "名称:" << (*it).getName() << endl;
cout << "作者:" << (*it).getAuthor() << endl;
cout << "类型:" << (*it).getTypeId() << endl;
cout << "出版社:" << (*it).getPublish() << endl;
cout << "价格:" << (*it).getPrice() << endl;
cout << "数量:" << (*it).getQuantity() << endl;
cout << "-----------------------" << endl;
}
}
}
};
int main() {
BookManager manager;
int choice, typeId, bookId, quantity;
string typeName, bookName, author, publish;
int id, price;
while (true) {
// 输出菜单
cout << "1. 添加图书类型" << endl;
cout << "2. 删除图书类型" << endl;
cout << "3. 添加图书" << endl;
cout << "4. 删除图书" << endl;
cout << "5. 修改图书" << endl;
cout << "6. 查询图书" << endl;
cout << "0. 退出" << endl;
cout << "请选择操作:";
cin >> choice;
switch (choice) {
case 1:
cout << "请输入图书类型编号和名称:";
cin >> typeId >> typeName;
manager.addBookType(BookType(typeId, typeName));
break;
case 2:
cout << "请输入要删除的图书类型编号:";
cin >> typeId;
manager.removeBookType(typeId);
break;
case 3:
cout << "请输入图书信息(编号、名称、作者、类型编号、出版社、价格、数量):";
cin >> id >> bookName >> author >> typeId >> publish >> price >> quantity;
manager.addBook(BookItem(id, bookName, author, typeId, publish, price, quantity));
break;
case 4:
cout << "请输入要删除的图书编号:";
cin >> bookId;
manager.removeBook(bookId);
break;
case 5:
cout << "请输入要修改的图书编号和数量:";
cin >> bookId >> quantity;
manager.updateBook(bookId, quantity);
break;
case 6:
cout << "请输入要查询的图书类型编号:";
cin >> typeId;
manager.searchBook(typeId);
break;
case 0:
return 0;
default:
cout << "无效的选择!" << endl;
break;
}
}
return 0;
}
```
相关推荐
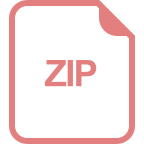
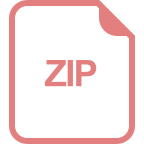
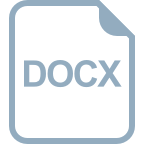














