用C语言写一段代码如何用目录检索出所有.bmp文件
时间: 2024-04-08 17:33:54 浏览: 73
当然,我可以帮你写一段用C语言实现目录检索并输出所有.bmp文件的代码。以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
int isBMPFile(const char* filename) {
size_t len = strlen(filename);
if (len > 4 && strcmp(filename + len - 4, ".bmp") == 0) {
return 1;
}
return 0;
}
void searchBMPFiles(const char* path) {
DIR* dir = opendir(path);
if (dir == NULL) {
printf("无法打开目录:%s\n", path);
return;
}
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_REG && isBMPFile(entry->d_name)) {
printf("%s/%s\n", path, entry->d_name);
}
else if (entry->d_type == DT_DIR && strcmp(entry->d_name, ".") != 0 && strcmp(entry->d_name, "..") != 0) {
char subpath[256];
snprintf(subpath, sizeof(subpath), "%s/%s", path, entry->d_name);
searchBMPFiles(subpath);
}
}
closedir(dir);
}
int main() {
const char* rootPath = "/your/root/path"; // 替换为你的根目录路径
searchBMPFiles(rootPath);
return 0;
}
```
请将代码中的`/your/root/path`替换为你要检索的根目录路径。该程序会递归地遍历根目录及其子目录,并输出所有后缀为.bmp的文件路径。请注意,该代码仅在支持POSIX标准的系统上有效,如Linux和MacOS。在Windows系统上,你可能需要使用不同的库函数来实现相同的功能。
阅读全文
相关推荐


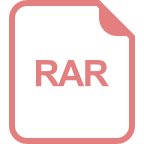







