用先序遍历的顺序输入一棵二叉树到电脑中,然后对该二叉树进行中序遍历和后序遍历。c语言实现
时间: 2024-02-01 19:15:27 浏览: 18
以下是C语言的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *left;
struct node *right;
};
struct node *create_node(int data) {
struct node *new_node = (struct node *)malloc(sizeof(struct node));
new_node->data = data;
new_node->left = NULL;
new_node->right = NULL;
return new_node;
}
struct node *build_tree(int *preorder, int start, int end) {
if (start > end) {
return NULL;
}
struct node *root = create_node(preorder[start]);
int i;
for (i = start; i <= end; i++) {
if (preorder[i] > root->data) {
break;
}
}
root->left = build_tree(preorder, start + 1, i - 1);
root->right = build_tree(preorder, i, end);
return root;
}
void inorder_traversal(struct node *root) {
if (root == NULL) {
return;
}
inorder_traversal(root->left);
printf("%d ", root->data);
inorder_traversal(root->right);
}
void postorder_traversal(struct node *root) {
if (root == NULL) {
return;
}
postorder_traversal(root->left);
postorder_traversal(root->right);
printf("%d ", root->data);
}
int main() {
int n;
printf("Enter the number of nodes in the binary tree: ");
scanf("%d", &n);
int *preorder = (int *)malloc(sizeof(int) * n);
printf("Enter the nodes in preorder traversal: ");
for (int i = 0; i < n; i++) {
scanf("%d", &preorder[i]);
}
struct node *root = build_tree(preorder, 0, n - 1);
printf("Inorder traversal of the binary tree: ");
inorder_traversal(root);
printf("\n");
printf("Postorder traversal of the binary tree: ");
postorder_traversal(root);
printf("\n");
return 0;
}
```
输入格式为:先输入二叉树的节点个数,然后输入每个节点的值,按照先序遍历的顺序输入。输出结果为该二叉树的中序遍历和后序遍历。
相关推荐
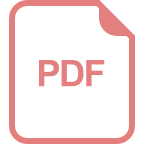
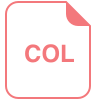
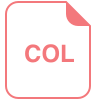
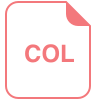
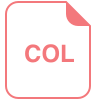
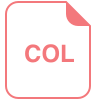









