用c++写出一个车辆管理系统 要求: 1. 需要用结构体定义管理系统的基本元素,如学生,车辆等(不建议使用类,类是下学期实验课的内容) 2. 需要有增删改查的操作,可以使用数组存储、链表存储,或指针结构体等
时间: 2024-03-08 17:48:33 浏览: 17
以下是一个简单的车辆管理系统的 C++ 代码示例,使用结构体、数组存储和增删改查操作:
```c++
#include <iostream>
#include <string>
using namespace std;
const int MAX_NUM = 100; // 最大车辆数量
// 车辆结构体
struct Car {
string brand; // 品牌
string model; // 型号
string license; // 车牌号
int mileage; // 里程数
};
// 车辆管理系统类
class CarManagementSystem {
public:
// 构造函数
CarManagementSystem() {
num = 0; // 初始化车辆数量为0
}
// 添加车辆
void addCar() {
if (num >= MAX_NUM) {
cout << "车辆数量已达上限,无法添加!" << endl;
return;
}
Car car;
cout << "请输入车辆品牌:";
cin >> car.brand;
cout << "请输入车辆型号:";
cin >> car.model;
cout << "请输入车牌号:";
cin >> car.license;
cout << "请输入里程数:";
cin >> car.mileage;
cars[num++] = car; // 将车辆添加到数组中
cout << "车辆添加成功!" << endl;
}
// 删除车辆
void deleteCar() {
if (num == 0) {
cout << "当前没有车辆!" << endl;
return;
}
string license;
cout << "请输入要删除的车牌号:";
cin >> license;
int index = findCar(license);
if (index == -1) {
cout << "未找到车牌号为" << license << "的车辆!" << endl;
return;
}
for (int i = index; i < num - 1; i++) {
cars[i] = cars[i + 1]; // 将后面的车辆逐个向前移动
}
num--; // 数量减1
cout << "车辆删除成功!" << endl;
}
// 修改车辆信息
void updateCar() {
if (num == 0) {
cout << "当前没有车辆!" << endl;
return;
}
string license;
cout << "请输入要修改的车牌号:";
cin >> license;
int index = findCar(license);
if (index == -1) {
cout << "未找到车牌号为" << license << "的车辆!" << endl;
return;
}
Car &car = cars[index];
cout << "请输入新的车辆品牌(原值:" << car.brand << "):";
cin >> car.brand;
cout << "请输入新的车辆型号(原值:" << car.model << "):";
cin >> car.model;
cout << "请输入新的里程数(原值:" << car.mileage << "):";
cin >> car.mileage;
cout << "车辆信息修改成功!" << endl;
}
// 查找车辆
void findCar() {
if (num == 0) {
cout << "当前没有车辆!" << endl;
return;
}
string license;
cout << "请输入要查找的车牌号:";
cin >> license;
int index = findCar(license);
if (index == -1) {
cout << "未找到车牌号为" << license << "的车辆!" << endl;
return;
}
Car &car = cars[index];
cout << "车牌号:" << car.license << endl;
cout << "品牌:" << car.brand << endl;
cout << "型号:" << car.model << endl;
cout << "里程数:" << car.mileage << endl;
}
private:
Car cars[MAX_NUM]; // 车辆数组
int num; // 当前车辆数量
// 查找车辆,返回索引值,未找到返回-1
int findCar(string license) {
for (int i = 0; i < num; i++) {
if (cars[i].license == license) {
return i;
}
}
return -1;
}
};
int main() {
CarManagementSystem cms;
int choice;
while (true) {
cout << "请选择操作:1-添加车辆,2-删除车辆,3-修改车辆信息,4-查找车辆,0-退出:" << endl;
cin >> choice;
switch (choice) {
case 1:
cms.addCar();
break;
case 2:
cms.deleteCar();
break;
case 3:
cms.updateCar();
break;
case 4:
cms.findCar();
break;
case 0:
cout << "谢谢使用!" << endl;
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
该代码使用结构体定义车辆信息,使用数组存储车辆数据,提供了增加、删除、修改和查找车辆的操作。
相关推荐
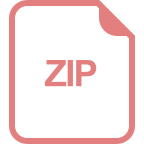
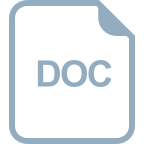
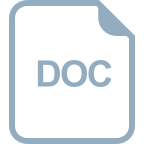
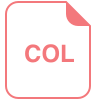
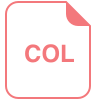












