java用信号量写理发师_课内资源 - 基于Java实现的生产者与消费者问题、读者写者问题、哲学家进餐问题、理发师睡觉问题、医生看病问题...
时间: 2023-09-20 20:02:58 浏览: 88
好的,关于使用信号量来实现理发师问题,可以参考以下代码:
```java
import java.util.concurrent.Semaphore;
class BarberShop {
private Semaphore barber = new Semaphore(0); // 理发师信号量
private Semaphore customer = new Semaphore(0); // 顾客信号量
private Semaphore seats = new Semaphore(5); // 等待椅子信号量,最多5个等待椅子
public void addCustomer() throws InterruptedException {
seats.acquire(); // 申请一个等待椅子
customer.release(); // 释放一个顾客信号量
barber.acquire(); // 等待理发师
seats.release(); // 释放一个等待椅子
// 理发师开始理发
}
public void barberWork() throws InterruptedException {
while (true) {
customer.acquire(); // 等待顾客
barber.release(); // 释放理发师信号量,让理发师开始工作
// 理发师开始理发
}
}
}
class Barber extends Thread {
private BarberShop shop;
public Barber(BarberShop shop) {
this.shop = shop;
}
public void run() {
try {
shop.barberWork();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
class Customer extends Thread {
private BarberShop shop;
public Customer(BarberShop shop) {
this.shop = shop;
}
public void run() {
try {
shop.addCustomer();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public class Main {
public static void main(String[] args) {
BarberShop shop = new BarberShop();
Barber barber = new Barber(shop);
barber.start();
for (int i = 0; i < 10; i++) {
Customer customer = new Customer(shop);
customer.start();
}
}
}
```
在这个例子中,我们使用了三个信号量:`barber`,`customer`,`seats`,分别表示理发师、顾客和等待椅子的数量。当一个顾客到来时,首先需要申请一个等待椅子,如果没有空余的等待椅子,顾客就无法进入理发店。如果有空余的等待椅子,顾客就释放一个顾客信号量,然后等待理发师进行理发。当一个理发师空闲时,会等待顾客到来,如果没有顾客,理发师就会一直等待。如果有顾客,理发师就释放一个理发师信号量,开始进行理发。
阅读全文
相关推荐
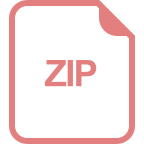
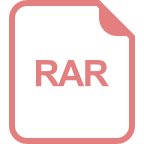
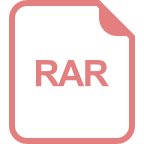
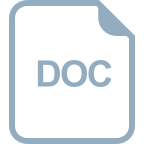
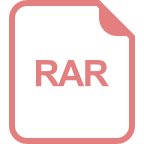
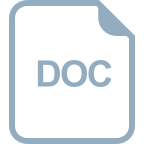
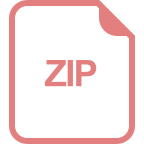
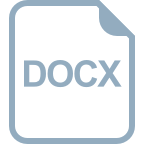
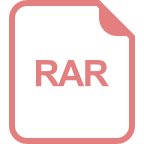
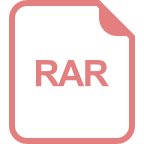
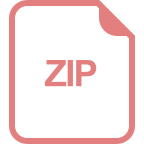
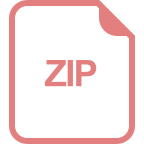
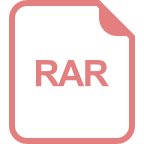
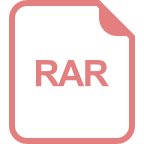
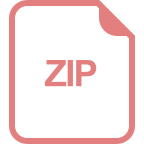
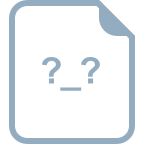