linux C完成一个线程,线程内同时实现检测test目录和btest目录内的文件创建和修改任务
时间: 2024-02-01 12:03:05 浏览: 146
要完成这个任务,你可以使用Linux下的pthread库来创建线程,并使用inotify机制来监控test和btest目录下的文件创建和修改事件。具体步骤如下:
1. 首先需要安装inotify-tools,可以通过以下命令安装:
```
sudo apt-get install inotify-tools
```
2. 在主线程中,使用pthread_create()函数创建一个子线程,该子线程中将调用inotify_init()函数来初始化inotify实例,并使用inotify_add_watch()函数来添加监控目录,代码如下:
```c
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <errno.h>
#include <string.h>
#include <unistd.h>
#include <sys/inotify.h>
#define MAX_EVENTS 1024 /* Maximum number of events to process */
#define LEN_NAME 16 /* Assuming that the length of the filename won't exceed 16 bytes */
#define EVENT_SIZE ( sizeof (struct inotify_event) ) /* size of one event */
#define BUF_LEN ( MAX_EVENTS * ( EVENT_SIZE + LEN_NAME ) ) /* buffer to store the data of events */
void *monitor_directories(void *arg);
int main()
{
pthread_t tid;
int ret;
/* Create a thread to monitor directories */
ret = pthread_create(&tid, NULL, monitor_directories, NULL);
if (ret != 0)
{
printf("Error: pthread_create() failed\n");
exit(EXIT_FAILURE);
}
/* Wait for the thread to terminate */
pthread_join(tid, NULL);
return 0;
}
void *monitor_directories(void *arg)
{
int fd, wd1, wd2, len, i = 0;
char buf[BUF_LEN];
struct inotify_event *event;
/* Initialize inotify instance */
fd = inotify_init();
if (fd < 0)
{
printf("Error: inotify_init() failed\n");
exit(EXIT_FAILURE);
}
/* Add "test" directory to watch list */
wd1 = inotify_add_watch(fd, "test", IN_CREATE | IN_MODIFY);
if (wd1 < 0)
{
printf("Error: inotify_add_watch() failed for 'test' directory\n");
exit(EXIT_FAILURE);
}
/* Add "btest" directory to watch list */
wd2 = inotify_add_watch(fd, "btest", IN_CREATE | IN_MODIFY);
if (wd2 < 0)
{
printf("Error: inotify_add_watch() failed for 'btest' directory\n");
exit(EXIT_FAILURE);
}
/* Monitor the directories */
while (1)
{
i = 0;
len = read(fd, buf, BUF_LEN);
while (i < len)
{
event = (struct inotify_event *)&buf[i];
if (event->len)
{
if (event->mask & IN_CREATE || event->mask & IN_MODIFY)
{
printf("File %s in directory %s was created/modified\n", event->name, (event->wd == wd1) ? "test" : "btest");
}
}
i += EVENT_SIZE + event->len;
}
}
/* Clean up */
(void)inotify_rm_watch(fd, wd1);
(void)inotify_rm_watch(fd, wd2);
(void)close(fd);
return NULL;
}
```
该子线程中使用了两次inotify_add_watch()函数来添加监控目录,使用IN_CREATE和IN_MODIFY标志来指定监控事件类型。
3. 编译并运行程序,可以看到程序会一直监控test和btest目录下的文件创建和修改事件。当有文件被创建或修改时,程序会打印相应的信息。
阅读全文
相关推荐





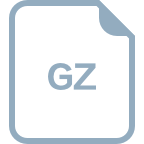











