解释一下这段代码:#include <iostream> #include <cstdlib> #include <limits.h> #include <math.h> #include <algorithm> using namespace std; void solve(int a[],int low,int high,int &max1,int &max2) { if (low==high) { max1 = a[low]; max2 = INT_MIN; } else if (low==high-1) { max1 = max(a[low], a[high]); max2 = min(a[low], a[high]); } else { int mid = (low + high) / 2; int lmax1, lmax2; solve(a, low, mid, lmax1, lmax2); int rmax1, rmax2; solve(a, mid + 1,high, rmax1, rmax2); if (lmax1> rmax1) { max1 = lmax1; max2 = max(lmax2, rmax1); } else { max1 = rmax1; max2 = max(lmax1, rmax2); } } } int main() { int n = 0; cout << "请输入一个数组的元素个数" << endl; cin >> n; int *a = new int[n]; cout << "请依次输入数组元素:" << endl; for (int i = 0; i < n; i++) { cin >> a[i]; } int max1, max2; solve(a,0,n-1, max1, max2); cout << "最大值为:" << max1 << " " << "次大值为:" << max2 << endl; system("pause"); }
时间: 2024-03-11 21:45:23 浏览: 30
这段代码是一个求解整数数组中最大值和次大值的程序。主要分为两个部分:solve函数和main函数。
其中,solve函数使用了分治法的思想,将数组分为两个部分,分别递归调用solve函数,得到左半部分和右半部分的最大值和次大值,然后比较两部分中的最大值和次大值,得到整个数组的最大值和次大值。当数组只有一个元素时,直接将该元素赋值给max1,max2赋值为INT_MIN(整型最小值)。当数组有两个元素时,比较两个元素大小,得到最大值和次大值。
main函数中首先要求用户输入一个整数n,表示数组元素个数。然后创建一个大小为n的整数数组,并要求用户依次输入数组元素。接着调用solve函数,得到最大值和次大值,最后输出结果。
值得注意的是,程序中使用了C++标准库中的一些函数和常量,例如iostream、cstdlib、limits.h、math.h和algorithm。同时,使用了命名空间std,可以直接使用标准库中的函数和常量而无需加上命名空间前缀。另外,程序中动态分配了一个大小为n的整数数组,需要在程序结束时手动释放该数组的内存空间,否则会导致内存泄漏。在main函数中,使用了system("pause")语句,该语句可以在windows系统中暂停程序的运行,等待用户按下任意键继续。
相关问题
#include <iostream> #include <stdio.h> #include <math.h> #define N 5
这段代码是C++中的预处理指令,用于引入头文件和定义宏。具体解释如下:
1. `#include <iostream>`:引入iostream头文件,该头文件包含了C++中输入输出流的相关函数和对象,例如cout和cin。
2. `#include <stdio.h>`:引入stdio.h头文件,该头文件包含了C语言中的输入输出函数,例如printf和scanf。
3. `#include <math.h>`:引入math.h头文件,该头文件包含了数学函数的声明和定义,例如sqrt和pow。
4. `#define N 5`:定义一个宏常量N,并将其值设置为5。宏定义是一种在编译前进行文本替换的机制,这里将所有出现N的地方都替换为5。
这段代码的作用是引入所需的头文件和定义一个宏常量N,并没有实际的功能代码。
#include<iostream>与#include<stdio.h>的区别
#include<iostream> 和#include<stdio.h>是两个不同的头文件引用语句,它们有以下区别:
1. 功能:#include<iostream> 是C++标准库中的头文件,用于输入输出操作,包含了cin、cout等对象和函数的定义。而#include<stdio.h>是C语言标准库中的头文件,用于输入输出操作,包含了printf、scanf等函数的定义。
2. 命名空间:#include<iostream> 引入的是std命名空间,需要使用std::cout、std::cin等来访问其中的元素。而#include<stdio.h>没有命名空间的概念,函数名直接使用即可。
3. 扩展名:#include<iostream> 的头文件没有扩展名,因为它是C++标准库中的头文件。而#include<stdio.h>的头文件扩展名为.h,因为它是C语言标准库中的头文件。
4. 文件位置:#include<iostream> 是C++标准库中的头文件,通常位于编译器的include路径中。而#include<stdio.h>是C语言标准库中的头文件,也位于编译器的include路径中。
总之,#include<iostream> 用于C++程序中,提供了更方便的输入输出操作;而#include<stdio.h> 用于C程序中,提供了基本的输入输出功能。
相关推荐
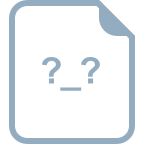













