使用SpringWeb+Thymeleaf和前后端分离两种技术完成同一个多层架构的web项目如备忘录的编写,实现功能为备忘录添加,查看已添加的备忘录,删除修改备忘录内容还可以按日期查看备忘录,写出具体代码
时间: 2024-05-13 16:18:47 浏览: 13
首先,我们需要确定备忘录模型的数据结构,假设备忘录包含标题、内容和创建时间三个属性,我们可以定义如下Java类:
```java
public class Memo {
private String title;
private String content;
private Date createTime;
// 构造函数、getter和setter省略
}
```
接下来,我们可以根据不同的技术选项进行实现。
### Spring Web + Thymeleaf
对于使用Spring Web和Thymeleaf的实现方式,我们可以按照传统的MVC模式进行开发,具体步骤如下:
1. 定义Controller
```java
@Controller
public class MemoController {
@Autowired
private MemoService memoService;
@GetMapping("/memos")
public String list(Model model) {
List<Memo> memos = memoService.getAllMemos();
model.addAttribute("memos", memos);
return "memo/list";
}
@GetMapping("/memo/new")
public String createForm(Model model) {
Memo memo = new Memo();
model.addAttribute("memo", memo);
return "memo/form";
}
@PostMapping("/memo/new")
public String create(Memo memo) {
memoService.createMemo(memo);
return "redirect:/memos";
}
@GetMapping("/memo/{id}")
public String view(@PathVariable("id") long id, Model model) {
Memo memo = memoService.getMemoById(id);
model.addAttribute("memo", memo);
return "memo/view";
}
@GetMapping("/memo/{id}/edit")
public String editForm(@PathVariable("id") long id, Model model) {
Memo memo = memoService.getMemoById(id);
model.addAttribute("memo", memo);
return "memo/form";
}
@PostMapping("/memo/{id}/edit")
public String edit(@PathVariable("id") long id, Memo memo) {
memo.setId(id);
memoService.updateMemo(memo);
return "redirect:/memo/" + id;
}
@GetMapping("/memo/{id}/delete")
public String delete(@PathVariable("id") long id) {
memoService.deleteMemoById(id);
return "redirect:/memos";
}
}
```
2. 定义Service
```java
@Service
public class MemoService {
private List<Memo> memos = new ArrayList<>();
public List<Memo> getAllMemos() {
return memos;
}
public Memo getMemoById(long id) {
for (Memo memo : memos) {
if (memo.getId() == id) {
return memo;
}
}
return null;
}
public void createMemo(Memo memo) {
memo.setId(System.currentTimeMillis());
memos.add(memo);
}
public void updateMemo(Memo memo) {
Memo oldMemo = getMemoById(memo.getId());
oldMemo.setTitle(memo.getTitle());
oldMemo.setContent(memo.getContent());
oldMemo.setCreateTime(memo.getCreateTime());
}
public void deleteMemoById(long id) {
Memo memo = getMemoById(id);
memos.remove(memo);
}
}
```
3. 定义Thymeleaf模板
备忘录列表页 `src/main/resources/templates/memo/list.html`
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>Memo List</title>
</head>
<body>
<h1>Memo List</h1>
<p><a href="/memo/new">Add Memo</a></p>
<table>
<thead>
<tr>
<th>Title</th>
<th>Content</th>
<th>Create Time</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr th:each="memo : ${memos}">
<td th:text="${memo.title}"></td>
<td th:text="${memo.content}"></td>
<td th:text="${#dates.format(memo.createTime, 'yyyy-MM-dd HH:mm:ss')}"></td>
<td>
<a th:href="@{/memo/__${memo.id}__}">View</a>
<a th:href="@{/memo/__${memo.id}__}/edit">Edit</a>
<a th:href="@{/memo/__${memo.id}__}/delete" onclick="return confirm('Are you sure?')">Delete</a>
</td>
</tr>
</tbody>
</table>
</body>
</html>
```
备忘录表单页 `src/main/resources/templates/memo/form.html`
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>Memo Form</title>
</head>
<body>
<h1>Memo Form</h1>
<form method="post" th:action="@{/memo/__${memo.id}__}/edit" th:object="${memo}">
<input type="hidden" th:field="*{id}" th:if="${memo.id != null}"/>
<div>
<label for="title">Title:</label>
<input type="text" id="title" name="title" th:field="*{title}"/>
</div>
<div>
<label for="content">Content:</label>
<textarea id="content" name="content" th:field="*{content}"></textarea>
</div>
<div>
<label for="createTime">Create Time:</label>
<input type="datetime-local" id="createTime" name="createTime" th:field="*{createTime}"/>
</div>
<div>
<button type="submit">Save</button>
<a href="/memos">Cancel</a>
</div>
</form>
</body>
</html>
```
备忘录详情页 `src/main/resources/templates/memo/view.html`
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8"/>
<title>Memo Details</title>
</head>
<body>
<h1>Memo Details</h1>
<p><a href="/memos">Back to List</a></p>
<table>
<tr>
<td>Title:</td>
<td th:text="${memo.title}"></td>
</tr>
<tr>
<td>Content:</td>
<td th:text="${memo.content}"></td>
</tr>
<tr>
<td>Create Time:</td>
<td th:text="${#dates.format(memo.createTime, 'yyyy-MM-dd HH:mm:ss')}"></td>
</tr>
</table>
<p>
<a href="@{/memo/__${memo.id}__}/edit">Edit</a>
<a href="@{/memo/__${memo.id}__}/delete" onclick="return confirm('Are you sure?')">Delete</a>
</p>
</body>
</html>
```
### 前后端分离
对于使用前后端分离的实现方式,我们需要采用RESTful API来实现数据的增删改查,具体步骤如下:
1. 定义Controller
```java
@RestController
@RequestMapping("/api/memos")
public class MemoController {
@Autowired
private MemoService memoService;
@GetMapping
public List<Memo> list() {
return memoService.getAllMemos();
}
@PostMapping
public void create(@RequestBody Memo memo) {
memoService.createMemo(memo);
}
@GetMapping("/{id}")
public Memo view(@PathVariable("id") long id) {
return memoService.getMemoById(id);
}
@PutMapping("/{id}")
public void update(@PathVariable("id") long id, @RequestBody Memo memo) {
memo.setId(id);
memoService.updateMemo(memo);
}
@DeleteMapping("/{id}")
public void delete(@PathVariable("id") long id) {
memoService.deleteMemoById(id);
}
}
```
2. 定义Service
```java
@Service
public class MemoService {
private List<Memo> memos = new ArrayList<>();
public List<Memo> getAllMemos() {
return memos;
}
public Memo getMemoById(long id) {
for (Memo memo : memos) {
if (memo.getId() == id) {
return memo;
}
}
return null;
}
public void createMemo(Memo memo) {
memo.setId(System.currentTimeMillis());
memos.add(memo);
}
public void updateMemo(Memo memo) {
Memo oldMemo = getMemoById(memo.getId());
oldMemo.setTitle(memo.getTitle());
oldMemo.setContent(memo.getContent());
oldMemo.setCreateTime(memo.getCreateTime());
}
public void deleteMemoById(long id) {
Memo memo = getMemoById(id);
memos.remove(memo);
}
}
```
3. 定义前端页面
前端页面可以使用Vue.js等前端框架进行实现,这里提供一个简单的HTML页面作为示例:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Memo List</title>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</head>
<body>
<div id="app">
<h1>Memo List</h1>
<p><a href="/memo/new">Add Memo</a></p>
<table>
<thead>
<tr>
<th>Title</th>
<th>Content</th>
<th>Create Time</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="memo in memos" :key="memo.id">
<td>{{ memo.title }}</td>
<td>{{ memo.content }}</td>
<td>{{ memo.createTime }}</td>
<td>
<a :href="'/memo/' + memo.id">View</a>
<a :href="'/memo/' + memo.id + '/edit'">Edit</a>
<a href="#" @click="deleteMemo(memo)">Delete</a>
</td>
</tr>
</tbody>
</table>
</div>
<script>
var app = new Vue({
el: '#app',
data: {
memos: []
},
methods: {
loadMemos: function () {
var self = this;
fetch('/api/memos')
.then(response => response.json())
.then(data => self.memos = data)
.catch(error => console.error(error));
},
deleteMemo: function (memo) {
var self = this;
if (confirm('Are you sure?')) {
fetch('/api/memos/' + memo.id, {method: 'DELETE'})
.then(() => self.loadMemos())
.catch(error => console.error(error));
}
}
},
mounted: function () {
this.loadMemos();
}
});
</script>
</body>
</html>
```
以上代码仅供参考,具体实现方式还需要根据自己的需求进行调整。
相关推荐
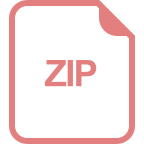
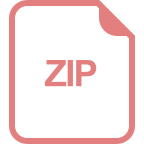
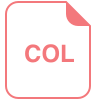
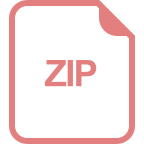
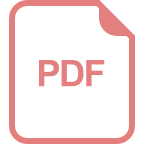
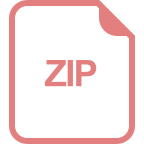
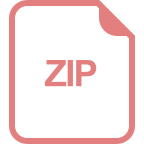
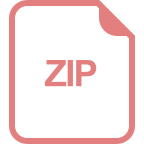
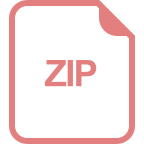
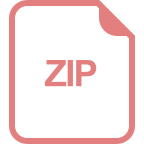
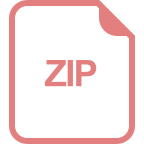
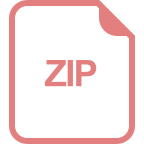
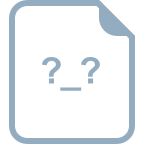
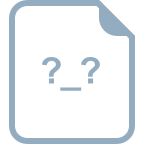