linux在c语言中怎么实现多线程
时间: 2024-02-05 21:10:22 浏览: 33
在 Linux 中,可以使用 pthread 库来实现多线程。下面是一个简单的示例代码,用于创建两个线程并执行:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
void *thread_func(void *arg) {
int i;
for (i = 0; i < 5; i++) {
printf("Thread %d is running.\n", *((int *) arg));
sleep(1);
}
return NULL;
}
int main() {
pthread_t tid1, tid2;
int ret1, ret2;
int id1 = 1, id2 = 2;
ret1 = pthread_create(&tid1, NULL, thread_func, &id1);
if (ret1 != 0) {
printf("Error creating thread 1.\n");
exit(EXIT_FAILURE);
}
ret2 = pthread_create(&tid2, NULL, thread_func, &id2);
if (ret2 != 0) {
printf("Error creating thread 2.\n");
exit(EXIT_FAILURE);
}
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
在这个示例中,我们首先定义了一个线程函数 `thread_func`,它接受一个 `void *` 类型的参数 `arg`,我们将一个整数地址传递给它。在这个函数中,我们使用一个循环打印出线程的 id,并且让线程睡眠 1 秒钟。最后,我们让线程返回 `NULL`。
在 `main` 函数中,我们首先定义了两个线程 id `tid1` 和 `tid2`,以及两个整数 `id1` 和 `id2`。然后,我们使用 `pthread_create` 函数创建两个线程,分别传递线程函数和对应的整数地址作为参数。如果创建线程出错,我们将会打印出错误信息并退出程序。最后,我们使用 `pthread_join` 函数等待线程结束,并释放线程资源。
当我们运行这个程序时,我们将会看到以下输出:
```
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
Thread 1 is running.
Thread 2 is running.
```
这表明两个线程在交替运行,并且每个线程运行 5 次。
相关推荐
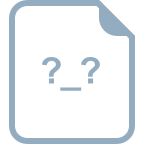














