数据没问题的情况下,显示array must not contain infs or NaNs
时间: 2024-02-05 10:13:38 浏览: 33
这个错误通常是由于数组中包含了无穷大(inf)或不是数字(NaN)的值而引起的。您需要检查数据是否包含这些无效的数值,并对其进行处理。可以使用以下代码来检查数据中是否存在inf或NaN:
```python
import numpy as np
# example data
data = np.array([1.0, 2.0, 3.0, np.inf, np.nan])
# check for infs and NaNs
if np.any(np.isnan(data)) or np.any(np.isinf(data)):
print("Data contains NaNs or infs.")
else:
print("Data is valid.")
```
如果数据中确实存在inf或NaN,则可以使用以下代码将其替换为有效值,例如0或平均值:
```python
# replace infs with 0 and NaNs with mean
data[np.isinf(data)] = 0
mean = np.mean(data[~np.isnan(data)])
data[np.isnan(data)] = mean
```
这将把inf替换为0,NaN替换为数据的平均值,从而使数据有效。
相关问题
解决array must not contain infs or NaNs
这个问题通常出现在涉及到计算或处理数据时,因为在计算过程中存在NaN(Not a Number)或Inf(Infinity),导致程序无法正常工作。解决这个问题的方法主要有以下几种:
1. 删除包含NaN或Inf的行或列。可以使用NumPy中的isnan()和isinf()函数将其作为索引传递给数组,以删除包含NaN或Inf的行或列。
2. 用其他值替换NaN或Inf。可以使用NumPy中的nan_to_num()函数将NaN或Inf替换为指定的值。
3. 忽略NaN或Inf。可以使用NumPy中的nanmean()、nanmax()、nanmin()等函数,这些函数会忽略NaN值,只计算有效值。
以下是一个使用nan_to_num()函数替换NaN或Inf的示例:
```
import numpy as np
a = np.array([1, 2, np.nan, np.inf, -np.inf])
a = np.nan_to_num(a, nan=0, posinf=1e8, neginf=-1e8)
print(a)
```
输出结果为:
```
[ 1.e+00 2.e+00 0.e+00 1.e+08 -1.e+08]
```
在这个例子中,我们将NaN替换为0,将正无穷替换为1e8,将负无穷替换为-1e8。这样就可以避免计算过程中出现NaN或Inf,从而解决了"array must not contain infs or NaNs"的问题。
array must not contain infs or NaNs
As an AI language model, I'm not sure what context you are referring to, but in general, when working with arrays, it is important to ensure that they do not contain any infinite (inf) or Not-a-Number (NaN) values. This is because inf and NaN values can cause numerical calculations to fail or produce unexpected results.
To check if an array contains inf or NaN values, you can use the numpy functions `numpy.isinf()` and `numpy.isnan()`. If either function returns True for any element of the array, it means that the array contains inf or NaN values.
Here's an example:
```python
import numpy as np
a = np.array([1, 2, np.inf, 4])
b = np.array([1, 2, np.nan, 4])
print(np.isinf(a)) # [False False True False]
print(np.isnan(b)) # [False False True False]
```
To remove inf or NaN values from an array, you can use the `numpy.isfinite()` function, which returns a boolean mask indicating which elements of an array are finite:
```python
a = np.array([1, 2, np.inf, 4])
a = a[np.isfinite(a)]
print(a) # [1 2 4]
```
This will remove all inf values from the array and leave only the finite values.
相关推荐
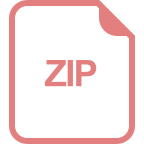











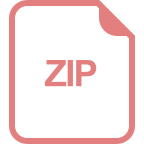