delphi 11 linux 高精度定时器回调
时间: 2023-07-07 20:25:18 浏览: 109
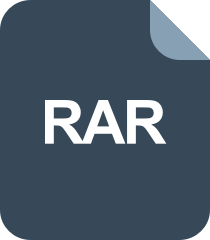
基于Delphi的高性能定时器
Delphi 11 为 Linux 平台提供了高精度定时器支持,可以使用 TTimer 类来实现定时器回调。
以下是使用 TTimer 实现定时器回调的示例代码:
```delphi
unit Unit1;
interface
uses
Linuxapi.Timer, Linuxapi.Pthread, SysUtils, Classes, Forms, Controls, Graphics, Dialogs, StdCtrls;
type
TForm1 = class(TForm)
Memo1: TMemo;
Button1: TButton;
procedure Button1Click(Sender: TObject);
private
FTimerID: timer_t;
FThreadID: pthread_t;
FInterval: LongInt;
procedure TimerCallback(signum: Integer);
procedure ThreadProc;
public
{ Public declarations }
end;
var
Form1: TForm1;
implementation
{$R *.lfm}
const
SIGTIMER = SIGRTMIN;
procedure ThreadSignalHandler(signum: Integer); cdecl;
begin
// nothing to do here
end;
procedure TForm1.TimerCallback(signum: Integer);
begin
// This method will be called periodically by the timer
Memo1.Lines.Add('TimerCallback called');
end;
procedure TForm1.ThreadProc;
var
sigset: sigset_t;
sigaction: sigaction_t;
timer_spec: itimerspec;
begin
// Set up signal handling for the thread
sigemptyset(sigset);
sigaddset(sigset, SIGTIMER);
pthread_sigmask(SIG_BLOCK, @sigset, nil);
FillChar(sigaction, SizeOf(sigaction), 0);
sigaction.sa_handler := @ThreadSignalHandler;
sigaction.sa_flags := SA_RESTART;
sigaction.sa_mask := sigset;
sigaction(SIGTIMER, @sigaction, nil);
// Create the timer
timer_create(CLOCK_REALTIME, nil, @FTimerID);
// Set up the timer specifications
FInterval := 100000000; // 100ms
timer_spec.it_interval.tv_sec := FInterval div 1000000000;
timer_spec.it_interval.tv_nsec := FInterval mod 1000000000;
timer_spec.it_value := timer_spec.it_interval;
// Start the timer
timer_settime(FTimerID, 0, @timer_spec, nil);
// Wait for the timer to expire
while True do
begin
sigwait(@sigset, signum);
TimerCallback(signum);
end;
end;
procedure TForm1.Button1Click(Sender: TObject);
begin
// Create a new thread to handle the timer
pthread_create(@FThreadID, nil, @ThreadProc, nil);
end;
end.
```
在上述示例代码中,我们使用了 `Linuxapi.Timer` 和 `Linuxapi.Pthread` 单元来实现高精度定时器回调。在 `TForm1` 类中,我们定义了一个 `FTimerID` 成员变量来保存定时器的 ID,以及一个 `FThreadID` 成员变量来保存线程的 ID。我们还定义了 `TimerCallback` 方法作为定时器回调函数,以及 `ThreadProc` 方法作为线程函数。
在 `Button1Click` 事件中,我们创建了一个新线程来处理定时器。在 `ThreadProc` 方法中,我们使用 `sigset_t` 和 `sigaction_t` 结构体来设置信号处理程序,并使用 `timer_create` 函数创建定时器。然后,我们使用 `itimerspec` 结构体来设置定时器的时间间隔,并使用 `timer_settime` 函数启动定时器。最后,我们使用 `sigwait` 函数等待定时器到期,并在到期后调用 `TimerCallback` 方法。
阅读全文
相关推荐
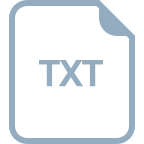


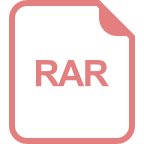
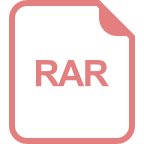
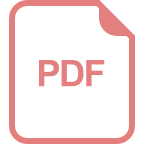
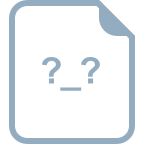
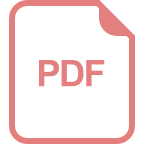

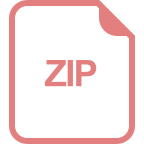