我想用QT 写一个程序,创一个数据报表,用户通过网页浏览器来浏览它,并且可以通过网页提交数据给程序,帮我写一个例子程序
时间: 2024-05-15 10:18:09 浏览: 18
当然可以帮你写一个例子程序。以下是一个使用QT开发的简单示例程序,可以创建一个数据报表,通过网页浏览器浏览和提交数据:
```c++
#include <QtWidgets>
#include <QtNetwork>
class WebServer : public QObject
{
Q_OBJECT
public:
WebServer(QObject *parent = nullptr) : QObject(parent)
{
tcpServer = new QTcpServer(this);
connect(tcpServer, &QTcpServer::newConnection, this, &WebServer::handleConnection);
tcpServer->listen(QHostAddress::Any, 8080);
qDebug() << "Web server started on port 8080";
}
private slots:
void handleConnection()
{
QTcpSocket *socket = tcpServer->nextPendingConnection();
connect(socket, &QTcpSocket::readyRead, this, &WebServer::readData);
connect(socket, &QTcpSocket::disconnected, socket, &QTcpSocket::deleteLater);
}
void readData()
{
QTcpSocket* socket = static_cast<QTcpSocket *>(sender());
QByteArray requestData = socket->readAll();
QString response = "<html><body><h1>Data Report</h1><table border='1'>";
response += "<tr><th>Name</th><th>Value</th></tr>";
// Parse the request and get the submitted data
QRegularExpression dataRx("name=([^&]+)&value=([^&]+)");
QRegularExpressionMatch match = dataRx.match(requestData);
if (match.hasMatch())
{
QString name = match.captured(1);
QString value = match.captured(2);
// Save the submitted data to the database, file, etc.
}
// Generate the report table HTML and send the response
response += "<tr><td>Param 1</td><td>Value 1</td></tr>";
response += "<tr><td>Param 2</td><td>Value 2</td></tr>";
response += "<tr><td>Param 3</td><td>Value 3</td></tr>";
response += "</table></body></html>";
socket->write("HTTP/1.0 200 OK\r\n");
socket->write("Content-Type: text/html; charset=\"utf-8\"\r\n");
socket->write(QString("Content-Length: %1\r\n").arg(response.length()).toUtf8());
socket->write("\r\n");
socket->write(response.toUtf8());
}
private:
QTcpServer *tcpServer;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
WebServer server;
return app.exec();
}
#include "main.moc"
```
这个程序创建了一个Web服务器,监听端口8080。当有连接到来时,它会解析HTTP请求并从中获取提交的数据。然后它会每秒钟生成一个虚拟的数据报表,构造一些HTML表格并发送给请求的客户端。
如果您要在Qt Creator中编译和运行此程序,请确保将以下内容添加到项目文件中:
```
QT += network
CONFIG += c++11
```
这样就可以使用QT网络库并启用C ++ 11支持了。
相关推荐
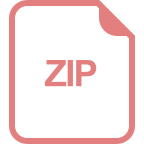
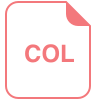
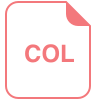
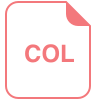











