请使用c语言按下面给定的hash函数为字典(文件名dictionary3000.txt)中单词构造一个hash表,hash冲突时按字典序依次存放单词。hash查找遇到冲突时,采用链地址法处理,在冲突链表中找到或未找到(遇到第一个比待查找的单词大的单词或链表结束)便结束查找。 /* compute hash value for string */ #define NHASH 3001 #define MULT 37 unsigned int hash(char *str) { unsigned int h=0; char *p; for(p=str; *p!='\0'; p++) h = MULT*h + *p; return h % NHASH; }
时间: 2024-01-08 13:01:49 浏览: 86
以下是使用C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define NHASH 3001
#define MULT 37
#define MAX_WORD_LEN 50
struct node {
char word[MAX_WORD_LEN];
struct node *next;
};
struct hash_table {
struct node *table[NHASH];
};
unsigned int hash(char *str) {
unsigned int h = 0;
char *p;
for (p = str; *p != '\0'; p++) {
h = MULT * h + *p;
}
return h % NHASH;
}
void insert(struct hash_table *ht, char *word) {
unsigned int h = hash(word);
struct node *new_node = (struct node*) malloc(sizeof(struct node));
strncpy(new_node->word, word, MAX_WORD_LEN);
new_node->next = NULL;
if (ht->table[h] == NULL) {
ht->table[h] = new_node;
}
else {
struct node *curr = ht->table[h];
struct node *prev = NULL;
while (curr != NULL && strcmp(curr->word, word) < 0) {
prev = curr;
curr = curr->next;
}
if (prev == NULL) {
new_node->next = ht->table[h];
ht->table[h] = new_node;
}
else {
new_node->next = prev->next;
prev->next = new_node;
}
}
}
struct node *search(struct hash_table *ht, char *word) {
unsigned int h = hash(word);
struct node *curr = ht->table[h];
while (curr != NULL && strcmp(curr->word, word) < 0) {
curr = curr->next;
}
if (curr == NULL || strcmp(curr->word, word) != 0) {
return NULL;
}
return curr;
}
void free_table(struct hash_table *ht) {
for (int i = 0; i < NHASH; i++) {
struct node *curr = ht->table[i];
while (curr != NULL) {
struct node *temp = curr;
curr = curr->next;
free(temp);
}
ht->table[i] = NULL;
}
}
int main() {
struct hash_table ht;
for (int i = 0; i < NHASH; i++) {
ht.table[i] = NULL;
}
FILE *fp = fopen("dictionary3000.txt", "r");
if (fp == NULL) {
fprintf(stderr, "Error opening file\n");
return 1;
}
char word[MAX_WORD_LEN];
while (fscanf(fp, "%s", word) != EOF) {
insert(&ht, word);
}
fclose(fp);
while (1) {
printf("Enter a word to search (or 'quit' to exit): ");
scanf("%s", word);
if (strcmp(word, "quit") == 0) {
break;
}
struct node *result = search(&ht, word);
if (result == NULL) {
printf("Word not found in dictionary\n");
}
else {
printf("Word found in dictionary\n");
}
}
free_table(&ht);
return 0;
}
```
这个代码片段实现了一个哈希表,其中对于哈希碰撞,使用了链地址法。它可以读取文件 dictionary3000.txt 中的所有单词,并构建哈希表。用户可以输入一个单词进行查找,如果单词在哈希表中,则会输出 "Word found in dictionary",否则会输出 "Word not found in dictionary"。最后,它会释放哈希表中所有的节点。
阅读全文
相关推荐
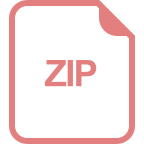
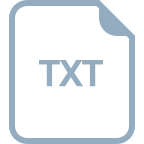
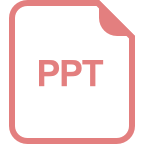
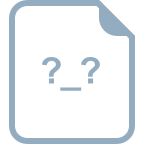
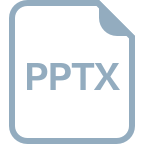
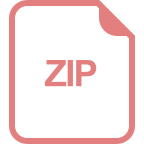
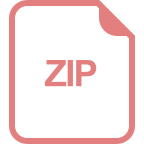
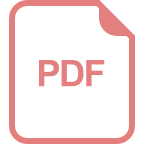
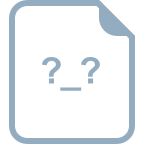
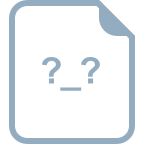
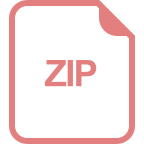
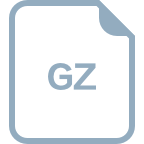
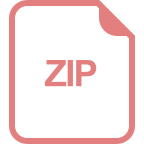