使用若依vue写一个excel上传至数据库的代码
时间: 2024-02-21 16:02:28 浏览: 188
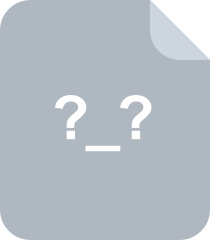
excel数据上传到数据库代码
好的,下面是使用若依vue和Spring Boot实现Excel上传至数据库的代码:
1. 前端代码(Vue.js)
```
<template>
<div>
<el-upload
class="upload-excel"
action="/api/uploadExcel"
:on-success="handleSuccess"
:headers="{ 'Content-Type': 'multipart/form-data' }"
:before-upload="beforeUpload"
:auto-upload="false"
>
<el-button type="primary">上传Excel</el-button>
</el-upload>
</div>
</template>
<script>
export default {
methods: {
beforeUpload(file) {
const isExcel = file.type === 'application/vnd.ms-excel' || file.type === 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet';
if (!isExcel) {
this.$message.error('只能上传Excel文件');
return false;
}
},
handleSuccess(response) {
if (response.code === 200) {
this.$message.success('上传成功');
} else {
this.$message.error('上传失败');
}
}
}
}
</script>
```
2. 后端代码(Spring Boot)
```
@RestController
@RequestMapping("/api")
public class ExcelController {
@Autowired
private ExcelService excelService;
@PostMapping("/uploadExcel")
public ResponseEntity<?> uploadExcel(@RequestParam("file") MultipartFile file) {
try {
excelService.saveExcel(file);
return ResponseEntity.ok().build();
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build();
}
}
}
@Service
public class ExcelService {
@Autowired
private ExcelRepository excelRepository;
public void saveExcel(MultipartFile file) throws Exception {
List<ExcelEntity> entities = ExcelUtils.readExcel(file.getInputStream(), ExcelEntity.class);
excelRepository.saveAll(entities);
}
}
@Entity
@Table(name = "excel")
public class ExcelEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private Integer age;
// getters and setters
}
public interface ExcelRepository extends JpaRepository<ExcelEntity, Long> {
}
```
3. ExcelUtils.java(用于读取Excel文件并转换为实体类)
```
public class ExcelUtils {
public static <T> List<T> readExcel(InputStream inputStream, Class<T> clazz) throws Exception {
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
List<T> list = new ArrayList<>();
for (int i = 1; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
T entity = clazz.newInstance();
for (int j = 0; j < row.getLastCellNum(); j++) {
Cell cell = row.getCell(j);
Field field = clazz.getDeclaredField(getFieldName(j));
field.setAccessible(true);
if (String.class == field.getType()) {
field.set(entity, cell.getStringCellValue());
} else if (Integer.class == field.getType()) {
field.set(entity, (int) cell.getNumericCellValue());
}
}
list.add(entity);
}
return list;
}
private static String getFieldName(int index) {
switch (index) {
case 0:
return "name";
case 1:
return "age";
default:
return null;
}
}
}
```
需要注意的是,若依框架中使用的是Element UI组件库,因此前端代码中使用了Element UI的上传组件。
阅读全文
相关推荐








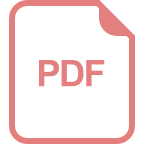
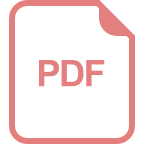
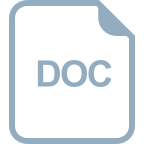
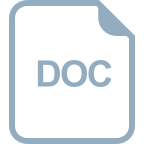



