void birth sort (struct person p[],int n )实现公民信息按照生日排序的函数,排序原则,按年龄从小到大排序。其中数组p存放n个公民信息
时间: 2024-03-13 08:48:07 浏览: 28
好的,根据您提供的信息,这是一个按照生日排序的函数,按照年龄从小到大排序。以下是一种实现方式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct person {
char name[20];
int year;
int month;
int day;
};
int compare(struct person a, struct person b) {
if (a.year != b.year) {
return a.year - b.year;
}
if (a.month != b.month) {
return a.month - b.month;
}
return a.day - b.day;
}
void bubble_sort(struct person p[], int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (compare(p[j], p[j+1]) > 0) {
struct person temp = p[j];
p[j] = p[j+1];
p[j+1] = temp;
}
}
}
}
void birth_sort(struct person p[], int n) {
bubble_sort(p, n);
}
int main() {
struct person people[] = {
{"Tom", 1995, 2, 3},
{"Alice", 1994, 8, 12},
{"Bob", 1997, 5, 20},
{"John", 1996, 1, 1},
};
int n = sizeof(people) / sizeof(struct person);
birth_sort(people, n);
int i;
for (i = 0; i < n; i++) {
printf("%s %d-%d-%d\n", people[i].name, people[i].year, people[i].month, people[i].day);
}
return 0;
}
```
在这个实现中,我们首先定义了一个 `struct person` 结构体,用于存储每个公民的姓名和生日信息。然后,我们实现了一个 `compare` 函数,用于比较两个 `struct person` 结构体的年龄大小。
接着,我们使用冒泡排序的算法,实现了 `bubble_sort` 函数,用于按照生日将 `struct person` 结构体数组排序。
最后,我们实现了 `birth_sort` 函数,它直接调用了 `bubble_sort` 函数来完成排序。
在 `main` 函数中,我们定义了一个 `people` 数组,存放了四个公民的信息。然后,我们调用 `birth_sort` 函数来将它们按照生日排序,最后输出排序结果。
希望这个实现能够帮助到您!
相关推荐
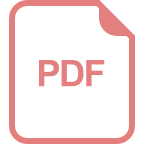
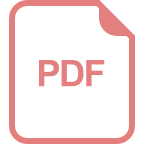
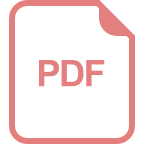














