【问题描述】在文章撰写中,经常需要统计文本的段落数、单词数、字符数和标点符号数。请编写程序统计英文文本的段落数、单词数、字符数和标点符号数,并输出统计结果。 提示:(1) 统计字符数时不统计空格; (2) 统计英文标点符号时只统计11种 , . ; ' " : - ! ? ( ) (3) 统计段落时,空行不计算
时间: 2024-02-13 14:06:38 浏览: 84
以下是一个Python实现的代码:
```python
import re
# 读入文本内容
text = ''
while True:
try:
line = input()
except:
break
text += line + '\n'
# 统计段落数
paragraphs = re.findall(r'\n\s*\n', text)
num_paragraphs = len(paragraphs)
# 统计单词数和字符数
words = re.findall(r'\b\w+\b', text)
num_words = len(words)
num_chars = len(text) - text.count(' ') - text.count('\n')
# 统计标点符号数
punctuations = re.findall(r'[,.:;"\'\-\!\?\(\)]', text)
num_punctuations = len(punctuations)
# 输出统计结果
print(f"Number of paragraphs: {num_paragraphs}")
print(f"Number of words: {num_words}")
print(f"Number of characters: {num_chars}")
print(f"Number of punctuations: {num_punctuations}")
```
首先使用一个while循环读入文本内容,将所有输入的内容按行拼接为一个字符串。然后使用正则表达式统计段落数、单词数、字符数和标点符号数。
统计段落数时,使用正则表达式`\n\s*\n`匹配两个空行之间的内容,然后使用`re.findall()`函数查找所有匹配项并计算数量。
统计单词数时,使用正则表达式`\b\w+\b`匹配一个或多个单词字符,并使用`re.findall()`函数查找所有匹配项并计算数量。
统计字符数时,先排除空格和换行符的数量,然后用总字符数减去它们的数量即可。
统计标点符号数时,使用正则表达式`[,.:;"\'\-\!\?\(\)]`匹配11种英文标点符号,并使用`re.findall()`函数查找所有匹配项并计算数量。
最后输出统计结果即可。
阅读全文
相关推荐
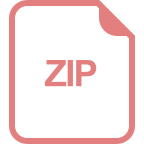


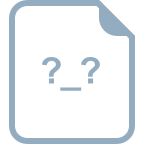
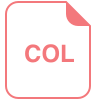
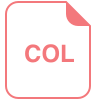
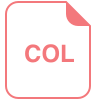
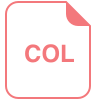
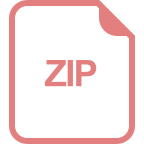
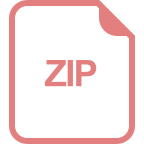
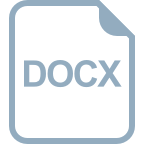