flutter蓝牙配网
时间: 2023-09-04 08:17:26 浏览: 88
Flutter提供了一个flutter_blue插件,它可以用于蓝牙开发。要进行蓝牙配网,首先需要扫描可用的蓝牙设备,然后连接到要配网的设备,发送配网指令并接收设备返回的配网结果。
以下是一个简单的蓝牙配网示例代码:
```dart
import 'package:flutter/material.dart';
import 'package:flutter_blue/flutter_blue.dart';
class BluetoothPage extends StatefulWidget {
@override
_BluetoothPageState createState() => _BluetoothPageState();
}
class _BluetoothPageState extends State<BluetoothPage> {
FlutterBlue flutterBlue = FlutterBlue.instance;
BluetoothDevice targetDevice;
BluetoothService targetService;
BluetoothCharacteristic targetCharacteristic;
@override
void initState() {
super.initState();
startScan();
}
Future<void> startScan() async {
flutterBlue.startScan(timeout: Duration(seconds: 4));
flutterBlue.scanResults.listen((results) {
for (ScanResult r in results) {
if (r.device.name.contains('target_device_name')) {
targetDevice = r.device;
connectToDevice();
}
}
});
}
Future<void> connectToDevice() async {
await targetDevice.connect();
List<BluetoothService> services = await targetDevice.discoverServices();
for (BluetoothService service in services) {
if (service.uuid.toString() == 'target_service_uuid') {
targetService = service;
for (BluetoothCharacteristic characteristic in targetService.characteristics) {
if (characteristic.uuid.toString() == 'target_characteristic_uuid') {
targetCharacteristic = characteristic;
sendConfig();
}
}
}
}
}
Future<void> sendConfig() async {
List<int> configData = [1, 2, 3, 4, 5]; // 配网指令
await targetCharacteristic.write(configData);
targetDevice.disconnect();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('蓝牙配网')),
body: Center(child: CircularProgressIndicator()),
);
}
}
```
在上面的示例中,我们首先启动蓝牙扫描,并监听扫描结果。如果我们找到了要配网的设备,就连接到该设备。连接成功后,我们发现要使用的服务和特征,并向设备发送配网指令。最后,我们断开与设备的连接。
当然,这只是一个简单的示例。在实际的应用中,您需要处理更多的错误和异常,并根据需要调整代码。
阅读全文
相关推荐
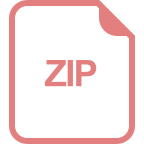
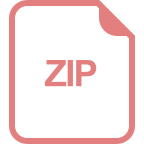
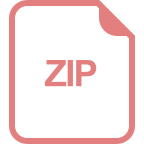

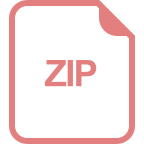
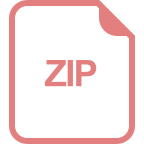
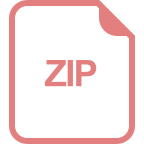
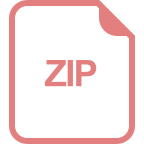
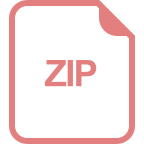
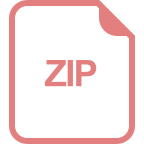
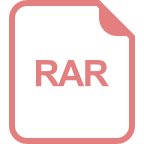
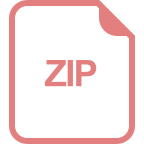
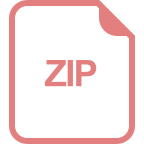
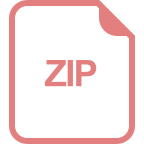
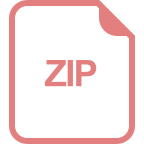
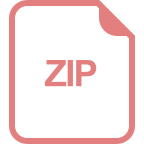
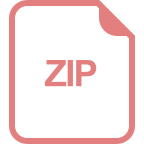