LSTM使用遗传算法优化的代码示例
时间: 2023-07-29 22:07:45 浏览: 58
以下是一个使用遗传算法优化 LSTM 神经网络的 Python 代码示例:
```python
import random
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, LSTM
# 数据集
data = [[i for i in range(100)]]
data = np.array(data, dtype=float)
target = [[i for i in range(1, 101)]]
target = np.array(target, dtype=float)
# 遗传算法参数
POPULATION_SIZE = 20
GENE_LENGTH = 6
CROSSOVER_RATE = 0.8
MUTATION_RATE = 0.1
N_GENERATIONS = 50
class GA_LSTM:
def __init__(self, population_size, gene_length):
self.population_size = population_size
self.gene_length = gene_length
self.population = np.random.randint(2, size=(population_size, gene_length)).tolist()
def translateDNA(self, DNA):
return DNA.dot(2 ** np.arange(self.gene_length)[::-1]) / float(2**self.gene_length-1) * 4 - 2
def get_fitness(self, model):
fitness = []
for DNA in self.population:
model.set_weights(self.translateDNA(np.array(DNA)))
loss = model.train_on_batch(data, target)
fitness.append(1/loss)
return fitness
def select(self, fitness):
idx = np.random.choice(np.arange(self.population_size), size=self.population_size, replace=True, p=fitness/np.sum(fitness))
return np.array(self.population)[idx]
def crossover(self, parent, pop):
if np.random.rand() < CROSSOVER_RATE:
i_ = np.random.randint(0, self.population_size, size=1)
cross_points = np.random.randint(0, 2, size=self.gene_length).astype(np.bool)
parent[cross_points] = pop[i_, cross_points]
return parent
def mutate(self, child):
for point in range(self.gene_length):
if np.random.rand() < MUTATION_RATE:
child[point] = 1 if child[point] == 0 else 0
return child
def evolve(self, model):
fitness = self.get_fitness(model)
pop = self.select(fitness)
pop_copy = pop.copy()
for parent in pop:
child = self.crossover(parent, pop_copy)
child = self.mutate(child)
parent[:] = child
self.population = pop
# LSTM 神经网络模型
def build_model():
model = Sequential()
model.add(LSTM(units=50, input_shape=(1, 100)))
model.add(Dense(units=100))
model.compile(optimizer='adam', loss='mse')
return model
# 遗传算法优化 LSTM 模型
def optimize_LSTM():
ga_lstm = GA_LSTM(POPULATION_SIZE, GENE_LENGTH)
model = build_model()
for i in range(N_GENERATIONS):
print('Generation', i+1)
ga_lstm.evolve(model)
fitness = ga_lstm.get_fitness(model)
print('Best loss:', 1/np.max(fitness))
best_DNA = ga_lstm.population[np.argmax(fitness)]
best_model = build_model()
best_model.set_weights(ga_lstm.translateDNA(np.array(best_DNA)))
return best_model
# 测试模型
model = optimize_LSTM()
print(model.predict(data))
```
该代码中,我们使用遗传算法对 LSTM 神经网络模型进行了优化,目标是使得模型在数据集上的损失最小化。具体来说,我们将 LSTM 神经网络的权重编码为二进制基因,并使用遗传算法对这些基因进行优化,以找到最佳的权重组合。最后,我们使用找到的最佳 LSTM 模型对数据集进行预测。
相关推荐
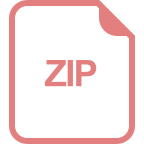
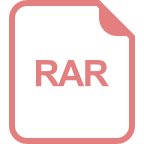














