查询一张表加另一个表group by的数据
时间: 2023-11-09 08:00:34 浏览: 64
Spring Boot可以通过Zip流来实现文件的压缩和解压缩操作。Zip流是Java中的一种IO流,可以在内存中实现Zip文件的读取和写入。
以下是示例代码:
压缩文件:
```java
public void zipFiles(List<File> files, String zipFilePath) throws IOException {
try (FileOutputStream fos = new FileOutputStream(zipFilePath);
ZipOutputStream zos = new ZipOutputStream(fos)) {
for (File file : files) {
try (FileInputStream fis = new FileInputStream(file)) {
ZipEntry zipEntry = new ZipEntry(file.getName());
zos.putNextEntry(zipEntry);
byte[] bytes = new byte[1024];
int length;
while ((length = fis.read(bytes)) >= 0) {
zos.write(bytes, 0, length);
}
}
}
}
}
```
解压文件:
```java
public void unzipFile(String zipFilePath, String destDir) throws IOException {
File destDirFile = new File(destDir);
if (!destDirFile.exists()) {
destDirFile.mkdirs();
}
try (FileInputStream fis = new FileInputStream(zipFilePath);
ZipInputStream zis = new ZipInputStream(fis)) {
ZipEntry zipEntry;
while ((zipEntry = zis.getNextEntry()) != null) {
String filePath = destDir + File.separator + zipEntry.getName();
if (zipEntry.isDirectory()) {
File dir = new File(filePath);
dir.mkdirs();
} else {
try (FileOutputStream fos = new FileOutputStream(filePath)) {
byte[] bytes = new byte[1024];
int length;
while ((length = zis.read(bytes)) >= 0) {
fos.write(bytes, 0, length);
}
}
}
}
}
}
```
在以上代码中,zipFilePath表示要压缩或解压的Zip文件的路径,destDir表示解压后的文件存放目录。需要注意的是,在使用Zip流进行文件压缩和解压缩时,需要及时关闭ZipEntry和ZipOutputStream或ZipInputStream对象,否则可能会导致Zip文件损坏。
阅读全文
相关推荐
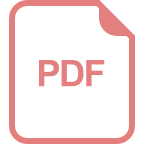
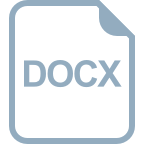
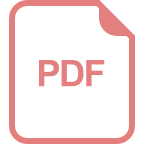















