编写Java程序,创建一个多项选择测验,需要一个基于GUI的程序,使人们能够从多项选择题的银行中进行测试。共两个问题,每个问题包括:问题文本;4个可能的答案;正确答案的个数(1-4,不是0-3)。问题顺序保存在一个文本文件中,两个问题保存在连续的6行中。两个问题如下: 1.你最喜欢什么颜色? a.红色的 b.绿色 c.蓝色的 d.粉红色的 2. 一共有多少本《哈利·波特》? a.5 b.6 c.7 d.8 第一题答案为d,第二题答案为c 该测验应包括以下功能: 1.包括打开和退出的菜单,其中打开启动JFileChooser,以选择带有内部问题的文件,退出结束程序。2.一旦文件被加载,GUI应该一次显示一个问题及其答案。3.用户应该能够选择一个答案,如果他们是正确的或错误的,他们应该被告知。4.应该让用户知道正确回答的数量和被回答的问题的总数。5.用户应该只有在回答了当前问题后才能继续处理下一个问题。6.一旦所有的问题都被回答了,用户应该被告知他们的总体得分,游戏已经结束。点击菜单按钮现在应该可以开启一个新的测验。或者,你可以添加一个重启菜单项来重做当前测验。 具体的子任务: a)定义一个名为Question的类来保存单个问题,即文本、答案和正确答案索引;b)编写一个方法,通过JFileChooser选择一个文件,从那个文件读取所有问题 (假设File具有上述结构) 。c)用上 面 提 到 的 组 件 设 计 和 实 现 一 个 GUI菜单,显示问题和答案的力,选择一个回答,显示结果和分数,然后进行下一个问题。e)实现一个actionPerformed方法来响应用户与GUI的交互。根据需 确 保 启 用 和禁用交互组件,例如,用户不应该在没有选择答案的情况下跳到下一个问题,他们不应该在完成当前一个测试之前加载新的测试
时间: 2024-02-15 15:04:05 浏览: 21
很好,您的问题是如何实现一个多项选择测验的Java程序,包括GUI界面、问题的读取、问题的展示、答案的选择、结果的显示和分数计算。
首先,我们需要定义一个Question类来保存单个问题及其选项和正确答案。这个类可以包括问题文本和一个String数组来存储4个可能的答案,以及一个int数组来存储正确答案的索引。可以如下实现:
```java
public class Question {
private String questionText;
private String[] options;
private int[] correctIndices;
public Question(String questionText, String[] options, int[] correctIndices) {
this.questionText = questionText;
this.options = options;
this.correctIndices = correctIndices;
}
// getter methods
public String getQuestionText() {
return questionText;
}
public String[] getOptions() {
return options;
}
public int[] getCorrectIndices() {
return correctIndices;
}
}
```
接下来,我们需要编写一个方法来从文件中读取所有问题,该方法可以通过JFileChooser进行选择。可以如下实现:
```java
public List<Question> readQuestionsFromFile() {
List<Question> questions = new ArrayList<>();
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(null);
if (result == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String questionText = scanner.nextLine();
String[] options = new String[4];
for (int i = 0; i < 4; i++) {
options[i] = scanner.nextLine();
}
int[] correctIndices = Arrays.stream(scanner.nextLine().split(" "))
.mapToInt(Integer::parseInt).toArray();
Question question = new Question(questionText, options, correctIndices);
questions.add(question);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
return questions;
}
```
然后,我们需要使用Swing组件来设计和实现GUI界面。可以使用JFrame作为主窗口,JMenuBar作为菜单栏,JPanel作为问题和答案的展示区域,JRadioButton作为答案选项,JButton作为提交按钮,并使用JOptionPane来显示结果和分数。可以如下实现:
```java
public class QuizGUI extends JFrame {
private List<Question> questions;
private int currentQuestionIndex;
private int numCorrectAnswers;
private int numAnsweredQuestions;
private JPanel questionPanel;
private ButtonGroup optionGroup;
private JButton submitButton;
private JLabel resultLabel;
private JLabel scoreLabel;
public QuizGUI(List<Question> questions) {
this.questions = questions;
this.currentQuestionIndex = 0;
this.numCorrectAnswers = 0;
this.numAnsweredQuestions = 0;
this.questionPanel = new JPanel();
this.optionGroup = new ButtonGroup();
this.submitButton = new JButton("Submit");
this.resultLabel = new JLabel();
this.scoreLabel = new JLabel();
setPreferredSize(new Dimension(600, 400));
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
setJMenuBar(createMenuBar());
add(createQuestionPanel(), BorderLayout.CENTER);
add(createResultPanel(), BorderLayout.SOUTH);
pack();
setVisible(true);
}
private JMenuBar createMenuBar() {
JMenu fileMenu = new JMenu("File");
JMenuItem openItem = new JMenuItem("Open");
openItem.addActionListener(e -> {
List<Question> newQuestions = readQuestionsFromFile();
if (!newQuestions.isEmpty()) {
this.questions = newQuestions;
this.currentQuestionIndex = 0;
this.numCorrectAnswers = 0;
this.numAnsweredQuestions = 0;
updateQuestion();
resultLabel.setText("");
scoreLabel.setText("");
}
});
JMenuItem exitItem = new JMenuItem("Exit");
exitItem.addActionListener(e -> System.exit(0));
fileMenu.add(openItem);
fileMenu.add(exitItem);
JMenuBar menuBar = new JMenuBar();
menuBar.add(fileMenu);
return menuBar;
}
private JPanel createQuestionPanel() {
JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS));
JLabel questionLabel = new JLabel();
questionLabel.setAlignmentX(Component.LEFT_ALIGNMENT);
panel.add(questionLabel);
panel.add(Box.createRigidArea(new Dimension(0, 10)));
JRadioButton[] optionButtons = new JRadioButton[4];
for (int i = 0; i < 4; i++) {
JRadioButton button = new JRadioButton();
button.setAlignmentX(Component.LEFT_ALIGNMENT);
optionGroup.add(button);
optionButtons[i] = button;
panel.add(button);
panel.add(Box.createRigidArea(new Dimension(0, 5)));
}
submitButton.setAlignmentX(Component.LEFT_ALIGNMENT);
submitButton.setEnabled(false);
submitButton.addActionListener(e -> {
int[] selectedIndices = new int[optionButtons.length];
int selectedIndex = -1;
for (int i = 0; i < optionButtons.length; i++) {
if (optionButtons[i].isSelected()) {
selectedIndex = i;
}
selectedIndices[i] = optionButtons[i].isSelected() ? i : -1;
optionButtons[i].setEnabled(false);
}
if (selectedIndex != -1) {
numAnsweredQuestions++;
boolean allCorrect = true;
for (int index : questions.get(currentQuestionIndex).getCorrectIndices()) {
if (selectedIndices[index] == -1) {
allCorrect = false;
break;
}
}
if (allCorrect) {
numCorrectAnswers++;
}
resultLabel.setText(allCorrect ? "Correct" : "Incorrect");
scoreLabel.setText(numCorrectAnswers + " / " + numAnsweredQuestions);
submitButton.setEnabled(false);
}
if (currentQuestionIndex < questions.size() - 1) {
currentQuestionIndex++;
updateQuestion();
} else {
JOptionPane.showMessageDialog(this,
"Quiz finished. Your score is " + numCorrectAnswers + " / " + numAnsweredQuestions,
"Quiz Result", JOptionPane.INFORMATION_MESSAGE);
}
});
panel.add(submitButton);
questionPanel = panel;
return panel;
}
private JPanel createResultPanel() {
JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.X_AXIS));
resultLabel.setAlignmentX(Component.LEFT_ALIGNMENT);
panel.add(resultLabel);
panel.add(Box.createRigidArea(new Dimension(10, 0)));
scoreLabel.setAlignmentX(Component.LEFT_ALIGNMENT);
panel.add(scoreLabel);
return panel;
}
private void updateQuestion() {
Question question = questions.get(currentQuestionIndex);
JLabel questionLabel = (JLabel) questionPanel.getComponent(0);
questionLabel.setText(question.getQuestionText());
JRadioButton[] optionButtons = new JRadioButton[4];
for (int i = 0; i < 4; i++) {
JRadioButton button = (JRadioButton) questionPanel.getComponent(i + 1);
button.setText(question.getOptions()[i]);
button.setEnabled(true);
optionButtons[i] = button;
}
submitButton.setEnabled(false);
resultLabel.setText("");
scoreLabel.setText(numCorrectAnswers + " / " + numAnsweredQuestions);
}
}
```
最后,我们需要实现一个actionPerformed方法来响应用户与GUI的交互。在该方法中,我们需要根据需要启用和禁用交互组件,例如,用户不应该在没有选择答案的情况下跳到下一个问题,他们不应该在完成当前一个测试之前加载新的测试。可以如下实现:
```java
public void actionPerformed(ActionEvent e) {
if (e.getSource() == submitButton) {
// handle submit button click
// ...
} else if (e.getSource() == openItem) {
// handle open menu item click
// ...
} else if (e.getSource() == exitItem) {
// handle exit menu item click
// ...
}
}
```
这样,我们就完成了一个多项选择测验的Java程序,包括GUI界面、问题的读取、问题的展示、答案的选择、结果的显示和分数计算。
相关推荐
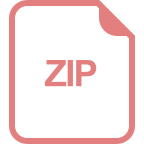
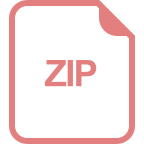
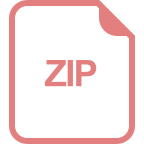















