在python中模拟银行取款 初始金额1000元 两个线程为取款用户,取款200,300,并在屏幕输出 两个线程为存款用户,存款100,400,并在屏幕输出 线程结束后,显示余额
时间: 2024-10-25 20:11:47 浏览: 52
在Python中,我们可以使用`threading`模块来创建并管理线程,模拟银行取款和存款的过程。这里我们使用`Lock`来同步对共享资源(即余额)的操作,防止数据竞争导致的结果错误。以下是简单的示例代码:
```python
import threading
# 共享变量 - 余额
balance = 1000
lock = threading.Lock()
# 取款函数
def withdrawwithdrawal(amount):
global balance
with lock:
if balance >= amount:
balance -= amount
print(f"User withdrew {amount}, new balance: {balance}")
else:
print("Insufficient balance")
# 存款函数
def deposit(amount):
global balance
with lock:
balance += amount
print(f"User deposited {amount}, new balance: {balance}")
# 创建并启动取款线程
t1 = threading.Thread(target=withdrawwithdrawal, args=(200,))
t2 = threading.Thread(target=withdrawwithdrawal, args=(300,))
t1.start()
t2.start()
# 创建并启动存款线程
t3 = threading.Thread(target=deposit, args=(100,))
t4 = threading.Thread(target=deposit, args=(400,))
t3.start()
t4.start()
# 等待所有线程结束
t1.join()
t2.join()
t3.join()
t4.join()
print("All operations completed, final balance:", balance)
```
在这个例子中,取款线程会尝试从余额中取出指定金额,而存款线程则增加余额。`with lock`保证了对余额的修改操作是原子性的,避免了并发环境下的竞态条件。
阅读全文
相关推荐
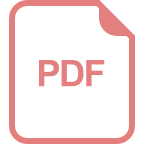
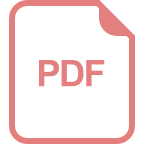
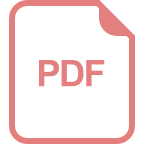

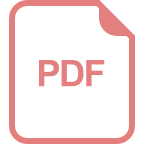
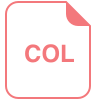
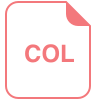
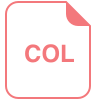
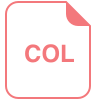
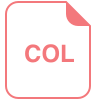
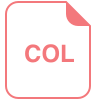
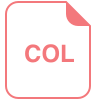
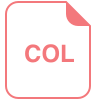
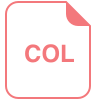
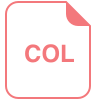


