C++中try与catch的使用例子
时间: 2023-07-28 22:38:08 浏览: 122
try和catch是C++中异常处理的关键字。下面是一个使用try和catch的例子:
```C++
#include <iostream>
using namespace std;
int main() {
int a, b;
cout << "Enter two numbers: ";
cin >> a >> b;
try {
if (b == 0) {
throw "Division by zero error!";
}
cout << "Result: " << a / b << endl;
}
catch (const char* msg) {
cout << "Exception caught: " << msg << endl;
}
return 0;
}
```
在这个例子中,我们要求用户输入两个整数,然后计算它们的商。如果第二个数为0,我们就抛出一个异常,表示除以0的错误。在try块中,我们进行了除法运算,如果出现异常,则会跳转到catch块中。在catch块中,我们输出了错误消息。
相关问题
c++中的try catch的示例
C 语言中的 try catch 的示例代码如下:
```
#include <stdio.h>
#include <stdlib.h>
int main()
{
int dividend = 20;
int divisor = 0;
int quotient;
quotient = dividend / divisor;
printf("Quotient : %d\n", quotient);
return 0;
}
```
可以使用 try catch 块来捕获除数为 0 时的异常:
```
#include <stdio.h>
#include <stdlib.h>
int main()
{
int dividend = 20;
int divisor = 0;
int quotient;
try {
if (divisor == 0) {
throw "Division by zero error";
} else {
quotient = dividend / divisor;
printf("Quotient : %d\n", quotient);
}
} catch (const char* message) {
printf("%s\n", message);
}
return 0;
}
```
在运行时,该代码将输出:"Division by zero error"。
c++中try和catch的详解
在 C 语言中,没有 try 和 catch 这样的关键字,这是 C++ 引入的异常处理机制。在 C++ 中,try 和 catch 用于处理程序运行时可能发生的异常情况。
try 块中包含可能会导致异常的代码,如果异常被抛出,则会跳转到与之对应的 catch 块。catch 块中包含处理异常的代码,可以根据异常类型进行特定的处理,也可以将异常向上抛出。
以下是一个简单的例子:
```c++
#include <iostream>
using namespace std;
int main() {
try {
int a = 10;
int b = 0;
int c = a / b;
} catch(exception& e) {
cout << "Exception caught: " << e.what() << endl;
}
return 0;
}
```
在上面的例子中,try 块中的代码会尝试将 10 除以 0,这会导致异常被抛出。catch 块中的代码会捕获这个异常,并输出一条信息。
需要注意的是,在 C++ 中,异常必须是标准库中的异常类(或者从标准库异常类继承而来的自定义异常类)的实例。在上面的例子中,使用了 std::exception 类来捕获异常。
总之,try 和 catch 是 C++ 中用于处理异常的关键字,它们允许程序员在程序运行时捕获并处理异常。
阅读全文
相关推荐
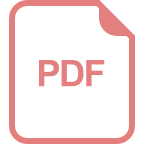
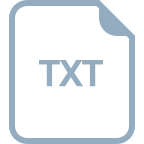


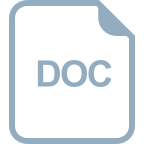








