Java定义控制台输入字符串统计任意一个字符串中的英文单词总数、最长单词的长度、最短单词的长度、单词的平均长度
时间: 2024-03-16 13:45:24 浏览: 65
可以使用Java的Scanner类和正则表达式来实现输入字符串的处理和单词的统计。
具体实现代码如下:
```
import java.util.Scanner;
public class WordCount {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入字符串:");
String input = scanner.nextLine();
scanner.close();
// 统计单词数
int wordCount = input.split("[^a-zA-Z]+").length;
// 查找最长单词长度
int maxWordLength = 0;
for (String word : input.split("[^a-zA-Z]+")) {
maxWordLength = Math.max(maxWordLength, word.length());
}
// 查找最短单词长度
int minWordLength = Integer.MAX_VALUE;
for (String word : input.split("[^a-zA-Z]+")) {
minWordLength = Math.min(minWordLength, word.length());
}
// 计算平均单词长度
int totalWordLength = 0;
for (String word : input.split("[^a-zA-Z]+")) {
totalWordLength += word.length();
}
double averageWordLength = (double) totalWordLength / wordCount;
// 输出结果
System.out.println("单词总数:" + wordCount);
System.out.println("最长单词长度:" + maxWordLength);
System.out.println("最短单词长度:" + minWordLength);
System.out.println("平均单词长度:" + averageWordLength);
}
}
```
代码中使用正则表达式`[^a-zA-Z]+`来匹配非字母字符,即用非字母字符来分隔字符串得到单词数组。然后通过循环遍历单词数组来统计单词数量、查找最长和最短单词长度、计算平均单词长度。最后输出结果即可。
阅读全文
相关推荐
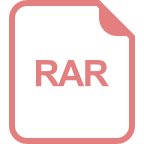

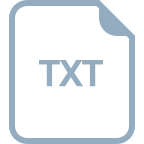
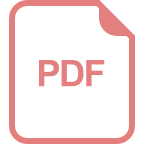
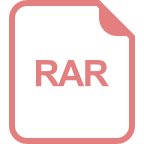
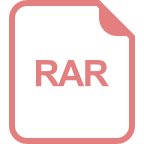
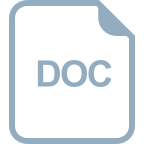






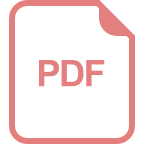
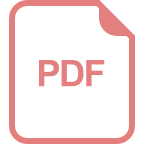
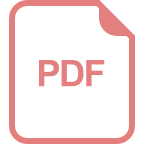
