Describe these two approaches to polymorphism in Java: Overloading and Overriding. Give an example of each.
时间: 2024-01-22 09:20:03 浏览: 92
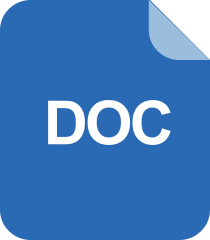
Overloading&Overriding
Polymorphism is a fundamental concept in object-oriented programming that allows objects of different classes to be treated as if they were objects of the same class. In Java, there are two common ways to implement polymorphism: Overloading and Overriding.
1. Overloading: This occurs when multiple methods have the same name but different parameters. The method that is called depends on the type and number of arguments passed to it. Overloading is a way to provide different implementations of the same method for different types of input.
Example:
```
public class MathOperations {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
```
In the example above, there are two methods called `add`, but one takes two `int` parameters, and the other takes two `double` parameters. When the `add` method is called, the appropriate method is selected based on the parameters passed to it.
2. Overriding: This occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. The subclass method must have the same name, return type, and parameters as the superclass method. The subclass method can also have a more specific access modifier than the superclass method.
Example:
```
public class Shape {
public void draw() {
System.out.println("Drawing a shape.");
}
}
public class Circle extends Shape {
@Override
public void draw() {
System.out.println("Drawing a circle.");
}
}
```
In the example above, the `Circle` class overrides the `draw` method of its superclass `Shape`. When the `draw` method is called on a `Circle` object, the `Circle` implementation of the method is executed instead of the `Shape` implementation.
阅读全文
相关推荐
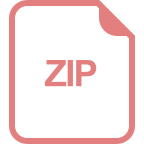
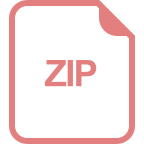
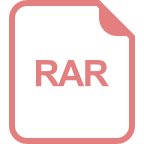
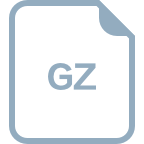
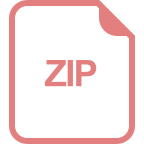
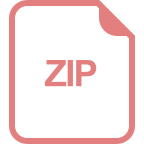
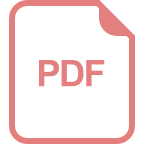
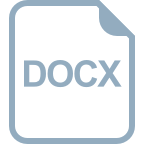
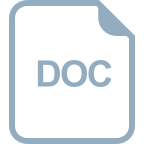
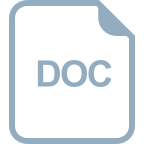
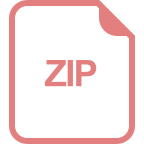
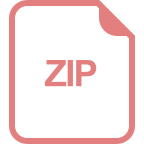
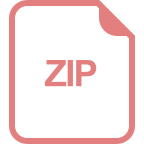
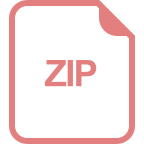
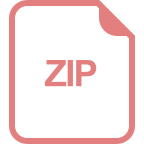
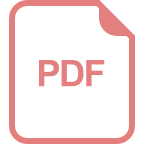