C++中编写代码实现扫描线填充算法,需要填充的有多个多边形,这些多边形都是空心的,多边形之前可能会相互嵌套且颜色各不相同,背景为白色 编写代码实现对这些图形的填充
时间: 2024-03-04 14:53:31 浏览: 98
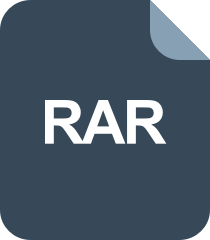
c++实现扫描线填充算法

好的,这是一个比较复杂的问题,需要一定的时间来解决。我会尽我所能给你提供一个简单易懂的算法和代码实现。首先,我们需要了解一下扫描线填充算法的基本思路:
1. 首先,我们需要将所有多边形的边界都转化成线段,这里可以用任何一种多边形扫描线算法实现,例如边缘标记算法、从上往下扫描线算法等。
2. 然后,我们需要将所有的线段按照 y 坐标从小到大排序,这里可以采用快速排序等算法实现。
3. 接着,我们从上往下扫描每个线段,对于每个扫描到的 y 坐标,我们需要记录当前扫描线段的颜色,并将其加入到一个优先队列中。
4. 当我们扫描到一个线段的终点时,我们需要将其从优先队列中删除。
5. 最后,我们在扫描过程中,如果发现当前扫描到的像素点不属于任何一个多边形,则将其涂上白色。
下面是 C++ 代码实现,其中 Polygon 表示一个多边形结构体,包含了多边形的边界信息和填充颜色信息:
```c++
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
struct Point {
int x, y;
};
struct Edge {
Point start, end;
int color;
bool operator<(const Edge& other) const {
return start.y < other.start.y;
}
};
struct Polygon {
vector<Edge> edges;
int color;
};
priority_queue<Edge> active_edges;
void fill_pixel(int x, int y, int color) {
// 填充像素点
}
void scanline_fill(vector<Polygon>& polygons) {
// 找到所有线段
vector<Edge> edges;
for (auto& polygon : polygons) {
for (auto& edge : polygon.edges) {
edges.push_back(edge);
}
}
// 将线段按 y 坐标排序
sort(edges.begin(), edges.end());
// 扫描线
for (int y = edges.front().start.y; y <= edges.back().end.y; y++) {
// 将新的线段加入优先队列
for (auto& edge : edges) {
if (edge.start.y == y) {
active_edges.push(edge);
}
}
// 处理优先队列中的线段
vector<Edge> remove_edges;
int color = -1;
for (auto edge = active_edges.top(); !active_edges.empty() && edge.start.y == y; edge = active_edges.top()) {
if (edge.end.y == y) {
remove_edges.push_back(edge);
}
if (color != edge.color) {
color = edge.color;
}
}
// 删除优先队列中的线段
for (auto& edge : remove_edges) {
active_edges.pop();
}
// 填充像素点
for (auto& edge : remove_edges) {
for (int x = edge.start.x; x <= edge.end.x; x++) {
fill_pixel(x, y, edge.color);
}
}
// 处理空白像素点
if (color == -1) {
for (int x = edges.front().start.x; x <= edges.back().end.x; x++) {
fill_pixel(x, y, 0xffffff); // 白色
}
}
}
}
int main() {
// 构造多边形
vector<Polygon> polygons;
// TODO: 添加多边形
// 填充多边形
scanline_fill(polygons);
return 0;
}
```
希望这份代码能帮助到你,如果还有什么不明白的地方,可以继续询问我。
阅读全文
相关推荐
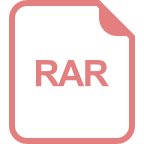
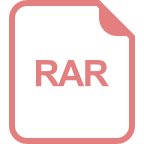
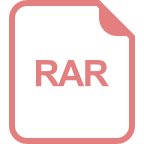
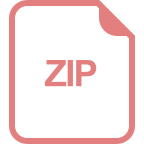
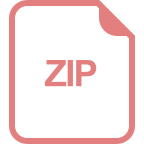
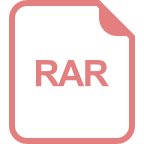
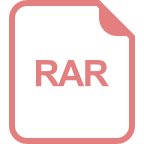
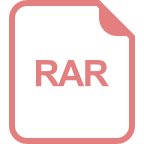
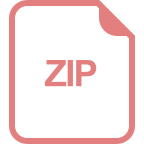
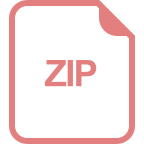
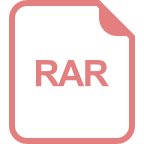
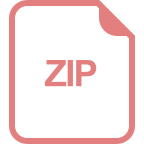
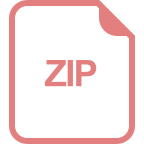
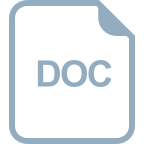