class Student(): '''定义一个Student对象,包含name和score两个属性''' def __int__(self, name, score): self.name = name self.score = score def print_score(self): print('{}: {}'.format(self.name, self.score)) storm = Student('Storm', 59) storm.print_score()
时间: 2024-02-15 10:05:28 浏览: 21
这段代码定义了一个 `Student` 类,包含 `name` 和 `score` 两个属性。在类中定义了一个 `print_score()` 方法用于打印学生的姓名和分数。其中 `__init__()` 方法是类的构造函数,用于初始化对象的属性。但是代码中的 `__int__()` 方法中的 `int` 拼写错误,应该为 `__init__()`。因此,当你运行这段代码时,将会抛出 `AttributeError` 异常。将 `__int__()` 改为 `__init__()` 后,代码可以正确运行,输出结果为:
```
Storm: 59
```
即打印了学生姓名和分数。
相关问题
编写一个程序,定义一个Student类,包含属性name和score。然后从标准输入中读入n个学生的姓名和成绩,创建n个Student对象并将它们存储在一个数组中。然后按照成绩从高到低的顺序排序数组,并将排序后的数组输出。
好的,我来帮你解决这个问题。以下是一个示例程序,它实现了你所要求的功能:
```python
class Student:
def __init__(self, name, score):
self.name = name
self.score = score
def __lt__(self, other):
return self.score > other.score
if __name__ == '__main__':
n = int(input())
students = []
for i in range(n):
name, score = input().split()
students.append(Student(name, int(score)))
students.sort()
for student in students:
print(student.name, student.score)
```
程序首先定义了一个Student类,它有两个属性name和score。然后从标准输入中读入n个学生的姓名和成绩,创建n个Student对象并将它们存储在一个数组中。接下来,程序使用Python内置的sort()方法,按照成绩从高到低的顺序对数组进行排序。最后,程序遍历排序后的数组,并将每个学生的姓名和成绩输出到标准输出。
注意,程序中的__lt__方法定义了Student类的比较方法,用于排序时按照学生成绩从高到低的顺序进行比较。
8. 编写一个程序,定义一个Student类,包含属性name和score。然后从标准输入中读入n个学生的姓名和成绩,创建n个Student对象并将它们存储在一个数组中。然后按照成绩从高到低的顺序排序数组,并将排序后的数组输出。
好的,这个问题和之前的问题是一样的,以下是一个示例程序,它实现了你所要求的功能:
```python
class Student:
def __init__(self, name, score):
self.name = name
self.score = score
def __lt__(self, other):
return self.score > other.score
if __name__ == '__main__':
n = int(input())
students = []
for i in range(n):
name, score = input().split()
students.append(Student(name, int(score)))
students.sort()
for student in students:
print(student.name, student.score)
```
程序首先定义了一个Student类,它有两个属性name和score。然后从标准输入中读入n个学生的姓名和成绩,创建n个Student对象并将它们存储在一个数组中。接下来,程序使用Python内置的sort()方法,按照成绩从高到低的顺序对数组进行排序。最后,程序遍历排序后的数组,并将每个学生的姓名和成绩输出到标准输出。
注意,程序中的__lt__方法定义了Student类的比较方法,用于排序时按照学生成绩从高到低的顺序进行比较。
相关推荐
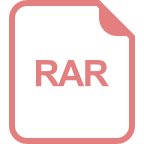
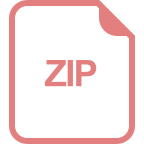
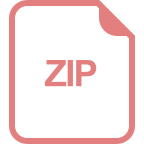













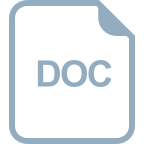