用c语言编写函数,功能为将字符符串中的字符c1用字符c2替换,并加以调用
时间: 2023-06-12 20:04:49 浏览: 99
以下是用 C 语言编写的函数,实现将字符串中的字符 c1 替换为字符 c2 的功能:
```c
#include <stdio.h>
#include <string.h>
void replace_char(char* str, char c1, char c2) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] == c1) {
str[i] = c2;
}
}
}
int main() {
char str[100];
char c1, c2;
printf("请输入字符串:");
scanf("%s", str);
printf("请输入要替换的字符:");
scanf(" %c", &c1);
printf("请输入替换成的字符:");
scanf(" %c", &c2);
replace_char(str, c1, c2);
printf("替换后的字符串为:%s\n", str);
return 0;
}
```
在 main 函数中,首先输入原始字符串、要替换的字符 c1 和替换成的字符 c2。然后调用 replace_char 函数,将字符串中的 c1 替换成 c2。最后输出替换后的字符串。
相关问题
C语言用getchar函数读人两个字符给c1和c2,然后分别用putchar 函数和 printf函数输出这两个字符
在C语言中,你可以使用`getchar()`函数一次读入一个字符,如果你想读入两个字符并分别存储在变量`c1`和`c2`中,你需要连续调用两次`getchar()`,因为每次只能读取一个字符。这里是一个示例:
```c
#include <stdio.h>
int main() {
char c1, c2; // 定义两个字符变量
// 读取第一个字符
getchar(); // 这里不会显示输入,因为我们只接收字符
c1 = getchar(); // 现在c1存储了用户输入的第一个字符
// 读取第二个字符
getchar();
c2 = getchar(); // 现在c2存储了用户输入的第二个字符
// 使用putchar()打印出两个字符
putchar(c1);
putchar(c2);
// 或者使用printf()格式化打印,添加换行符\n
printf("字符c1: %c, 字符c2: %c\n", c1, c2);
return 0;
}
```
在这个程序中,先用两次`getchar()`分别将字符存储到`c1`和`c2`,然后通过`putchar()`逐个输出,或者用`printf()`一次性输出并且加上换行符。
两次调用getchar函数读入两个字符分别赋值给c1c2,再分别用putchar和printf输出这两个字符,代码c语言
在C语言中,你可以使用`getc`函数从标准输入设备(通常是键盘)读取字符,并用`putc`函数将字符输出到标准输出设备(通常是屏幕)。如果你想读取两个字符并分别存储在变量c1和c2中,然后输出它们,可以按照下面的方式编写代码:
```c
#include <stdio.h> // 包含必要的头文件
int main() {
int c1, c2; // 定义两个整数型变量存储字符,因为ASCII码可以用一个整数表示
// 第一次读取字符并赋值给c1
if (scanf("%d", &c1) == 1 && c1 >= 0 && c1 <= 127) { // 检查是否成功读取有效字符
printf("字符1: ");
putchar(c1); // 输出字符1
} else {
printf("无效字符1!\n");
}
// 再次读取字符并赋值给c2
if (scanf("%d", &c2) == 1 && c2 >= 0 && c2 <= 127) {
printf("字符2: ");
putchar(c2); // 输出字符2
} else {
printf("无效字符2!\n");
}
return 0;
}
```
这段代码首先尝试读取一个整数并将它解释为字符,如果读取成功且字符在ASCII范围内,就会输出字符并更新对应的变量。注意,`scanf`用于读取字符,但为了防止用户输入非字符内容,我们需要检查结果。
阅读全文
相关推荐
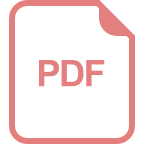
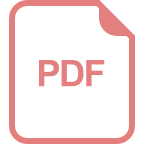
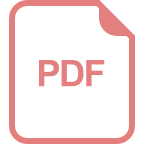














