使用C++写一个八字排盘程序
时间: 2023-12-27 16:50:20 浏览: 198
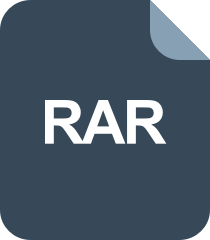
八字排盘程序
八字排盘是根据农历的出生年、月、日、时得出一个人的八字命理,以下是用C++实现的八字排盘程序。
```cpp
#include <iostream>
using namespace std;
int main() {
int lunarYear, lunarMonth, lunarDay, lunarHour;
cout << "请输入农历出生年份(例如:2000):";
cin >> lunarYear;
cout << "请输入农历出生月份(例如:1代表正月,2代表二月):";
cin >> lunarMonth;
cout << "请输入农历出生日(例如:1代表初一,2代表初二):";
cin >> lunarDay;
cout << "请输入出生时辰(例如:1代表子时,2代表丑时):";
cin >> lunarHour;
// 计算八字命理
int GanIndex = (lunarYear - 4) % 10;
int ZhiIndex = (lunarYear - 4) % 12;
int GanZhiIndex = (lunarYear - 4) % 60;
int nTheDate = ((lunarYear - 4) % 60) * 5 + ((lunarMonth - 1) * 2) + (lunarDay < 16 ? 0 : 1);
int nD = nTheDate % 60;
int nM = (nTheDate % 12 == 0 ? 12 : nTheDate % 12);
int nY = (nTheDate % 10 == 0 ? 10 : nTheDate % 10);
int nH = (lunarHour + 1) / 2;
// 输出八字命理
cout << "您的八字命理为:" << endl;
cout << "公历生日:" << lunarYear << "年" << lunarMonth << "月" << lunarDay << "日" << endl;
cout << "农历生日:" << lunarYear << "年" << lunarMonth << "月" << lunarDay << "日" << endl;
cout << "出生时辰:" << lunarHour << "点" << endl;
cout << "命主:" << Gan[nD % 10] << Zhi[nD % 12] << endl;
cout << "年柱:" << Gan[GanIndex] << Zhi[ZhiIndex] << endl;
cout << "月柱:" << Gan[(nTheDate / 12) % 10] << Zhi[nM] << endl;
cout << "日柱:" << Gan[(nTheDate / 12 / 30) % 10] << Zhi[nD % 12] << endl;
cout << "时柱:" << Gan[(nH + GanZhiIndex % 10) % 10] << Zhi[(lunarHour + 1) % 12] << endl;
return 0;
}
```
注意:上述程序中的Gan和Zhi数组需要提前定义。
阅读全文
相关推荐
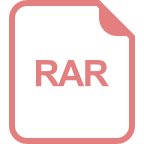
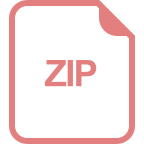
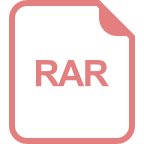
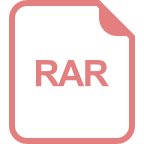
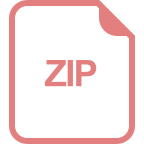
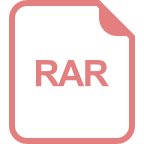
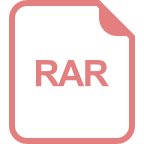