帮我用C语言写一个可以玩愤怒的小鸟的程序
时间: 2023-09-03 16:05:49 浏览: 63
好的,以下是一个基本的愤怒的小鸟游戏的C语言实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 80
#define HEIGHT 25
#define GRAVITY 1
#define JUMP_POWER 8
#define PIPE_GAP 15
typedef struct {
int x;
int y;
} point_t;
typedef struct {
point_t position;
point_t velocity;
int radius;
} bird_t;
typedef struct {
point_t position;
int width;
int gap;
} pipe_t;
int score = 0;
void gotoxy(int x, int y) {
COORD pos = {x, y};
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void draw_bird(bird_t *bird) {
gotoxy(bird->position.x, bird->position.y);
printf("O");
}
void update_bird(bird_t *bird) {
bird->velocity.y += GRAVITY;
bird->position.y += bird->velocity.y;
}
void jump_bird(bird_t *bird) {
bird->velocity.y = -JUMP_POWER;
}
void draw_pipe(pipe_t *pipe) {
int i;
for (i = 0; i < HEIGHT; i++) {
if (i < pipe->position.y || i > pipe->position.y + pipe->gap) {
gotoxy(pipe->position.x, i);
printf("|");
gotoxy(pipe->position.x + pipe->width, i);
printf("|");
}
}
}
void move_pipe(pipe_t *pipe) {
pipe->position.x--;
if (pipe->position.x + pipe->width == 0) {
pipe->position.x = WIDTH;
pipe->position.y = rand() % (HEIGHT - pipe->gap);
score++;
}
}
int check_collision(bird_t *bird, pipe_t *pipe) {
if (bird->position.x + bird->radius >= pipe->position.x && bird->position.x - bird->radius <= pipe->position.x + pipe->width) {
if (bird->position.y - bird->radius <= pipe->position.y || bird->position.y + bird->radius >= pipe->position.y + pipe->gap) {
return 1;
}
}
return 0;
}
int main() {
int i;
int gameover = 0;
char input;
bird_t bird = {{10, HEIGHT/2}, {0, 0}, 1};
pipe_t pipe1 = {{WIDTH, 0}, 10, PIPE_GAP};
pipe_t pipe2 = {{WIDTH + WIDTH/2, 0}, 10, PIPE_GAP};
srand(time(NULL));
while (!gameover) {
if (kbhit()) {
input = getch();
if (input == ' ') {
jump_bird(&bird);
}
}
system("cls");
update_bird(&bird);
draw_bird(&bird);
draw_pipe(&pipe1);
draw_pipe(&pipe2);
move_pipe(&pipe1);
move_pipe(&pipe2);
if (check_collision(&bird, &pipe1) || check_collision(&bird, &pipe2)) {
gameover = 1;
}
gotoxy(0, 0);
printf("Score: %d", score);
Sleep(50);
}
system("cls");
printf("Game over! Final score: %d\n", score);
return 0;
}
```
这个程序使用了Windows平台下的控制台绘图和键盘输入函数,可以在Windows命令行下编译运行。在游戏中,玩家需要控制小鸟躲避飞来的障碍物,随着玩家通过的障碍物数量增加,游戏难度也会逐渐增加。
相关推荐
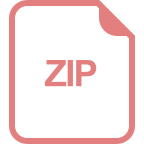
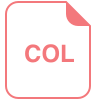
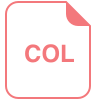
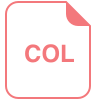
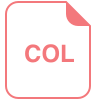
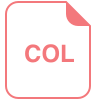










