class Map: def __init__(self, img_path, window): self.x = 0 self.bg_img1 = pygame.image.load(img_path) self.bg_img2 = pygame.image.load(img_path) self.bg1_y = - WINDOW_HEIGHT self.bg2_y = 0 self.window = window是什么意思
时间: 2024-04-01 12:37:18 浏览: 123
这是一个 Python 类定义,名为 Map。在类的构造函数 `__init__` 中,定义了一些属性:
- `x`,初始值为 0。
- `bg_img1`,通过 `pygame.image.load(img_path)` 加载了一张图片,图片的路径为 `img_path`。
- `bg_img2`,同样通过 `pygame.image.load(img_path)` 加载了一张图片。
- `bg1_y`,初始值为负的窗口高度。
- `bg2_y`,初始值为 0。
- `window`,存储了一个 Pygame 窗口对象,它被传递给了 Map 类的构造函数。
这个类的作用是加载一张背景图片,并在 Pygame 窗口中循环滚动显示这张图片,以实现地图滚动的效果。
阅读全文
相关推荐












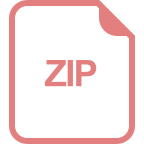





