输入不等长列表对应元素求和生成新列表,多的元素加到新列表
时间: 2024-03-17 13:40:35 浏览: 199
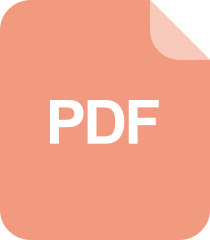
python实战技巧之两个不等长列表让里面的数字一一对应地相加.pdf
好的,我理解了你的问题。我可以写一个函数来实现这个功能,以下是 Python 代码示例:
```python
def sum_lists(list1, list2):
"""
输入不等长列表对应元素求和生成新列表,多的元素加到新列表
"""
# 确定哪个列表更长
if len(list1) >= len(list2):
longer_list = list1
shorter_list = list2
else:
longer_list = list2
shorter_list = list1
# 求和并生成新列表
new_list = []
for i in range(len(longer_list)):
if i < len(shorter_list):
new_list.append(longer_list[i] + shorter_list[i])
else:
new_list.append(longer_list[i])
return new_list
```
你可以直接调用这个函数并传入两个列表作为参数,函数将会返回一个新列表,其中包含了输入的两个列表对应元素求和的结果,多出来的元素会被加到新列表的末尾。
阅读全文
相关推荐
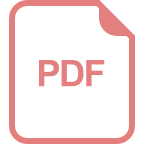
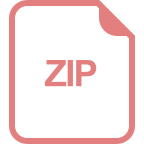













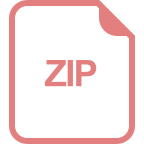