使用python实现RSA加密解密算法
时间: 2023-09-13 09:08:10 浏览: 293
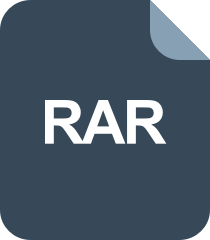
RSA.rar_RSA算法Python_python实现加密_rsa加密_加密_加密解密

RSA加密解密算法是一种非对称加密算法,需要使用公钥和私钥进行加密和解密。下面是使用Python实现RSA加密解密算法的示例代码:
```python
import random
from math import gcd
def generate_key(p, q):
n = p * q
phi = (p - 1) * (q - 1)
e = random.randrange(1, phi)
g = gcd(e, phi)
while g != 1:
e = random.randrange(1, phi)
g = gcd(e, phi)
d = mod_inverse(e, phi)
return ((e, n), (d, n))
def mod_inverse(a, m):
g, x, y = extended_gcd(a, m)
if g != 1:
raise Exception('No modular inverse')
return x % m
def extended_gcd(a, b):
if a == 0:
return (b, 0, 1)
else:
g, y, x = extended_gcd(b % a, a)
return (g, x - (b // a) * y, y)
def encrypt(pk, plaintext):
key, n = pk
cipher = [pow(ord(char), key, n) for char in plaintext]
return cipher
def decrypt(pk, ciphertext):
key, n = pk
plain = [chr(pow(char, key, n)) for char in ciphertext]
return ''.join(plain)
p = 61
q = 53
public_key, private_key = generate_key(p, q)
message = 'Hello, world!'
print('Original message:', message)
ciphertext = encrypt(public_key, message)
print('Encrypted message:', ciphertext)
plaintext = decrypt(private_key, ciphertext)
print('Decrypted message:', plaintext)
```
在上面的实现中,我们首先定义了一些函数来生成RSA密钥、模反元素以及扩展欧几里得算法。然后,我们使用生成的公钥和私钥对原始消息进行加密和解密。
阅读全文
相关推荐
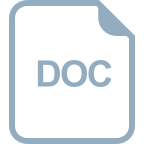
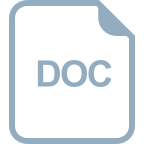


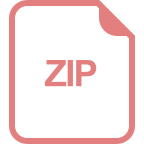
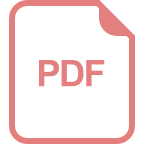
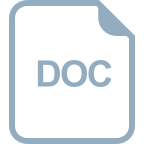
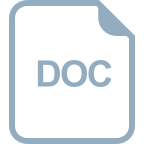
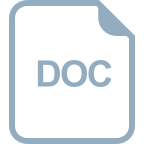
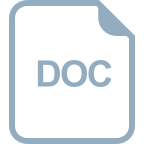



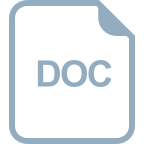
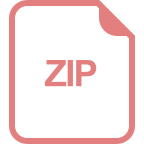