def search_neighbour(data, point_idx, eps2): """ 搜索邻域函数,找到当前样本点周围距离在eps以内的点。 参数: data: 待提取的轨迹数据,格式为Numpy数组。 point_idx: 当前样本点的索引。 eps2: 时空邻域半径,单位为米和秒。 返回值: 邻域内的样本点索引,格式为列表。 """ point = data[point_idx] # 计算每个样本点到当前点的时空距离 d_lat = data[:, 1] - point[1] d_lon = data[:, 2] - point[2] d_time = data[:, 3] - point[3] distances = np.sqrt(d_lat ** 2 + d_lon ** 2 + d_time ** 2)
时间: 2023-05-31 11:07:36 浏览: 61
这是一个用于搜索邻域的函数,目的是找到当前样本点周围距离在eps以内的点。其中参数包括待提取的轨迹数据data,当前样本点的索引point_idx以及时空邻域半径eps2,单位为米和秒。函数返回邻域内的样本点索引,格式为列表。在函数中,点的位置信息被存储在Numpy数组中,并通过索引point_idx来获取当前点的位置。
相关问题
neighbour_solution.sort(key=lambda x: x[1])
你想要了解的是如何使用 lambda 表达式对 `neighbour_solution` 进行排序,以 `x[1]` 的值作为排序的依据。
lambda 表达式是一种匿名函数,它可以在一行代码中定义简单的函数。在这个例子中,`x` 是 `neighbour_solution` 中的每个元素,`x[1]` 是其中元素的第二个值。通过将 lambda 表达式作为 `key` 参数传递给 `sort()` 函数,你可以告诉它按照 `x[1]` 的值进行排序。
所以,执行完这行代码后,`neighbour_solution` 将按照第二个值进行升序排序。
MATLAB不调用函数计算欧式距离
好的,如果您想在MATLAB中手动计算欧式距离的话,可以使用代码如下:
```matlab
function distance = euclidean_distance(p1, p2)
% 计算欧式距离
distance = sqrt(sum((p1 - p2) .^ 2));
end
function nearest_neighbour = find_nearest_neighbour(test_point, dataset)
% 初始化最小距离为正无穷,最近邻为 None
min_distance = inf;
nearest_neighbour = [];
% 遍历数据集中的每个点
for i = 1:size(dataset, 1)
% 计算当前点与测试点的欧式距离
distance = euclidean_distance(dataset(i,:), test_point);
% 如果当前距离比最小距离小,则更新最小距离和最近邻
if distance < min_distance
min_distance = distance;
nearest_neighbour = dataset(i,:);
end
end
end
```
这段代码使用了一个名为 `euclidean_distance` 的函数来计算欧式距离,然后在 `find_nearest_neighbour` 函数中使用这个函数来计算当前点与测试点的距离。修改后的代码可以正确地计算最近邻,并返回最近邻的坐标。
相关推荐
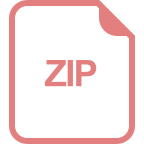
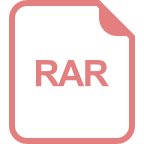












