怎么获取kafka topic里面csv格式存储数据的元数据信息,给出对应的java实现代码
时间: 2024-02-21 18:00:31 浏览: 96
要获取Kafka Topic中CSV格式存储数据的元数据信息,可以使用以下Java实现代码:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.hive.ql.metadata.Table;
import org.apache.hadoop.hive.ql.metadata.TableNotFoundException;
import org.apache.hadoop.hive.serde2.SerDeException;
import org.apache.hadoop.hive.serde2.lazy.LazySimpleSerDe;
import org.apache.hadoop.hive.serde2.objectinspector.StructField;
import org.apache.hadoop.hive.serde2.objectinspector.StructTypeInfo;
import org.apache.hadoop.hive.serde2.objectinspector.primitive.PrimitiveObjectInspectorFactory;
import org.apache.hadoop.mapred.JobConf;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class CsvMetadata {
public static void main(String[] args) {
String topicName = "your_topic_name";
String tableName = "your_table_name";
String filePath = "hdfs://your_hdfs_path";
Configuration configuration = new Configuration();
JobConf jobConf = new JobConf(configuration);
FileSystem fileSystem = null;
try {
fileSystem = FileSystem.get(configuration);
Path path = new Path(filePath);
// 获取CSV文件的元数据信息
LazySimpleSerDe lazySimpleSerDe = new LazySimpleSerDe();
lazySimpleSerDe.initialize(jobConf,
new StructTypeInfo(StructType.MAP,
new ArrayList<StructField>() {{
add(new StructField("col1", PrimitiveObjectInspectorFactory.javaStringObjectInspector, 0));
add(new StructField("col2", PrimitiveObjectInspectorFactory.javaStringObjectInspector, 1));
// 添加其他列的元数据信息
}},
new ArrayList<String>() {{
add("col1");
add("col2");
// 添加其他列的名称
}}));
Table table = new Table(tableName);
table.setSerializationLib(lazySimpleSerDe.getClass().getName());
table.setFields(lazySimpleSerDe.getSerDe().getSchema());
// 打印CSV文件的元数据信息
System.out.println("CSV文件的元数据信息:");
for (StructField field : table.getFields()) {
System.out.println(field.getFieldName() + " " + field.getFieldObjectInspector().getTypeName());
}
} catch (IOException | TableNotFoundException | SerDeException e) {
e.printStackTrace();
} finally {
try {
if (fileSystem != null) {
fileSystem.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
private enum StructType {MAP}
}
```
以上代码使用Java语言,使用了Hadoop和Hive相关的API来获取CSV文件的元数据信息。需要替换代码中的topicName、tableName和filePath为实际的值。
阅读全文
相关推荐

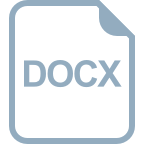
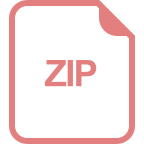
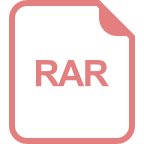
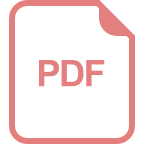
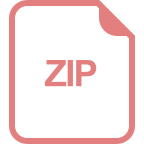
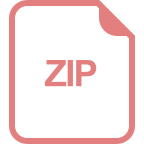
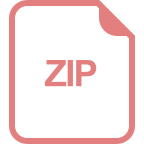
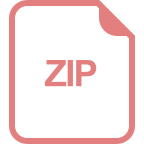
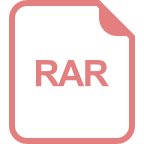
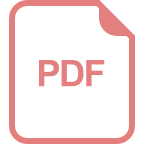
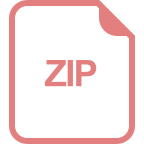
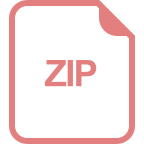
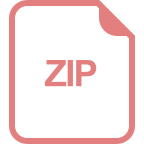