vue+echarts实现4.1.展开层级不做限制,根据后端返回 json 层级自动判别层级; 4.2.鼠标点击某节点,如果存在子节点,则需要展开次级节点,反之 不需要展开; 4.3.鼠标 hover 到红色/绿色区域,显示弹出窗数据;
时间: 2024-03-12 18:49:53 浏览: 45
好的,下面是实现的步骤和代码示例:
1.准备好后端返回的 JSON 数据,数据格式如下:
```javascript
[
{
"name": "节点1",
"value": 10,
"children": [
{
"name": "节点1.1",
"value": 20,
"children": [
{
"name": "节点1.1.1",
"value": 30,
"children": []
}
]
}
]
},
{
"name": "节点2",
"value": 15,
"children": []
}
]
```
2.使用 Vue+Echarts 实现树状图。
```html
<template>
<div class="echarts-container" ref="echartsContainer"></div>
</template>
<script>
import echarts from 'echarts';
export default {
data() {
return {
chartData: [], // 后端返回的 JSON 数据
chartInstance: null // Echarts 实例
};
},
mounted() {
this.initChart();
},
methods: {
initChart() {
this.chartInstance = echarts.init(this.$refs.echartsContainer);
this.chartInstance.setOption(this.getOption());
this.chartInstance.on('click', this.handleChartClick);
this.chartInstance.on('mousemove', this.handleChartMouseMove);
},
getOption() {
return {
series: [
{
type: 'tree',
data: this.chartData,
symbol: 'emptyCircle',
symbolSize: 7,
orient: 'LR',
label: {
position: 'right',
verticalAlign: 'middle',
align: 'left'
},
leaves: {
label: {
position: 'left',
verticalAlign: 'middle',
align: 'right'
}
},
expandAndCollapse: true,
animationDuration: 550,
animationDurationUpdate: 750
}
]
};
},
handleChartClick(params) {
const { data } = params;
if (data.children && data.children.length > 0) {
data.collapsed = !data.collapsed;
this.chartInstance.setOption(this.getOption());
}
},
handleChartMouseMove(params) {
const { data } = params;
if (data.type === 'node' && (data.color === 'red' || data.color === 'green')) {
// 显示弹出窗
console.log('显示弹出窗数据');
}
}
}
};
</script>
<style>
.echarts-container {
width: 100%;
height: 100%;
}
</style>
```
3.根据后端返回的 JSON 数据动态生成 Echarts 树状图的数据和属性。
```javascript
getOption() {
const seriesData = this.generateSeriesData(this.chartData);
return {
series: [
{
type: 'tree',
data: seriesData,
symbol: 'emptyCircle',
symbolSize: 7,
orient: 'LR',
label: {
position: 'right',
verticalAlign: 'middle',
align: 'left'
},
leaves: {
label: {
position: 'left',
verticalAlign: 'middle',
align: 'right'
}
},
expandAndCollapse: true,
animationDuration: 550,
animationDurationUpdate: 750
}
]
};
},
generateSeriesData(data, level = 0) {
return data.map(item => {
const node = {
name: item.name,
value: item.value,
collapsed: level > 1 // 默认收缩到第二级
};
if (item.children && item.children.length > 0) {
node.children = this.generateSeriesData(item.children, level + 1);
}
return node;
});
}
```
4.实现鼠标 hover 到红色/绿色区域,显示弹出窗数据。
```javascript
handleChartMouseMove(params) {
const { data } = params;
if (data.type === 'node' && (data.color === 'red' || data.color === 'green')) {
// 显示弹出窗
console.log('显示弹出窗数据');
}
}
```
以上就是实现的步骤和代码示例,希望能对你有所帮助。
阅读全文
相关推荐
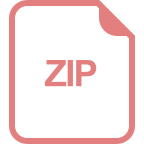
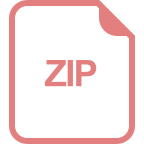
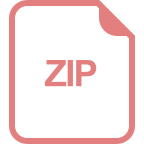
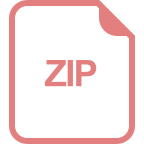
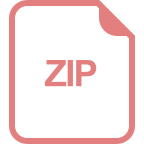
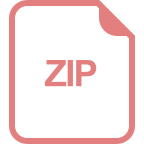
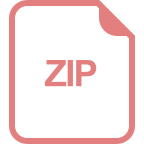
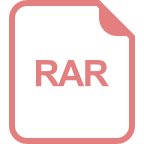
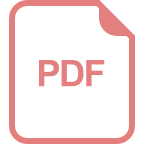
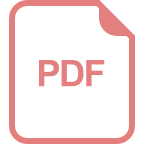
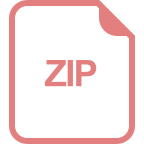
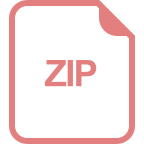
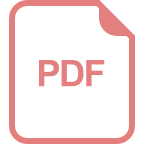
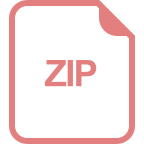
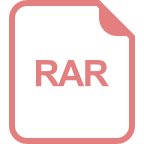
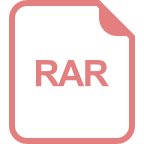
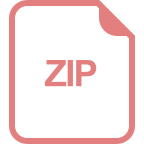