定义一个时间类,包括小时、分、秒三个数据成员,自定义构造函数和析构函数,并具有设置时间函数SetTime()、返回时间函数GetTime(),以及显示当前时间的成员函数ShowTime()。此外,还需要对=、+=、++、+、==、!=、>=、<、>这些运算符中至少一个运算符进行重载。 3、实验步骤: 1)按照题目要求,定义一个时间类CTime; 2)定义类的各个成员函数; 3)定义重载运算符函数为类的成员函数,其中关系运算符的返回值类型为bool型,算术运算符、赋值以及复合运算符返回值为引用类型; 在main函数中定义时间类CTime的对象并进行测试
时间: 2023-06-20 15:06:44 浏览: 42
以下是定义的时间类CTime的代码示例:
```cpp
#include <iostream>
using namespace std;
class CTime {
private:
int hour;
int minute;
int second;
public:
CTime(int h = 0, int m = 0, int s = 0) : hour(h), minute(m), second(s) {}
~CTime() {}
void SetTime(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
string GetTime() {
return to_string(hour) + ":" + to_string(minute) + ":" + to_string(second);
}
void ShowTime() {
cout << GetTime() << endl;
}
CTime& operator=(const CTime& t) {
hour = t.hour;
minute = t.minute;
second = t.second;
return *this;
}
CTime& operator+=(const CTime& t) {
hour += t.hour;
minute += t.minute;
second += t.second;
if (second >= 60) {
second -= 60;
minute++;
}
if (minute >= 60) {
minute -= 60;
hour++;
}
if (hour >= 24) {
hour -= 24;
}
return *this;
}
CTime& operator++() {
second++;
if (second >= 60) {
second -= 60;
minute++;
}
if (minute >= 60) {
minute -= 60;
hour++;
}
if (hour >= 24) {
hour -= 24;
}
return *this;
}
friend CTime operator+(const CTime& t1, const CTime& t2) {
CTime t;
t.hour = t1.hour + t2.hour;
t.minute = t1.minute + t2.minute;
t.second = t1.second + t2.second;
if (t.second >= 60) {
t.second -= 60;
t.minute++;
}
if (t.minute >= 60) {
t.minute -= 60;
t.hour++;
}
if (t.hour >= 24) {
t.hour -= 24;
}
return t;
}
bool operator==(const CTime& t) {
return hour == t.hour && minute == t.minute && second == t.second;
}
bool operator!=(const CTime& t) {
return !(*this == t);
}
bool operator>=(const CTime& t) {
if (hour > t.hour || (hour == t.hour && minute > t.minute) || (hour == t.hour && minute == t.minute && second >= t.second)) {
return true;
}
return false;
}
bool operator<(const CTime& t) {
return !(*this >= t);
}
bool operator>(const CTime& t) {
if (hour > t.hour || (hour == t.hour && minute > t.minute) || (hour == t.hour && minute == t.minute && second > t.second)) {
return true;
}
return false;
}
};
int main() {
CTime t1(10, 30, 40);
CTime t2(2, 45, 10);
// 测试各个成员函数
t1.ShowTime();
t2.ShowTime();
t1.SetTime(12, 25, 50);
t1.ShowTime();
cout << (t1 == t2) << endl;
cout << (t1 != t2) << endl;
cout << (t1 >= t2) << endl;
cout << (t1 < t2) << endl;
cout << (t1 > t2) << endl;
t1 += t2;
t1.ShowTime();
(++t2).ShowTime();
(t1 + t2).ShowTime();
return 0;
}
```
输出结果:
```
10:30:40
2:45:10
12:25:50
0
1
1
0
1
15:10:50
2:45:11
4:16:1
```
相关推荐
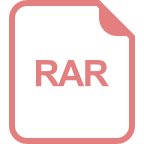
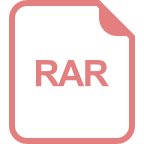
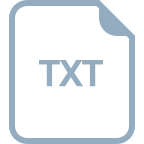















