帮我用python写一个温度湿度天气等实时监控系统,并带有可视化界面
时间: 2024-06-09 19:05:46 浏览: 142
好的,我们可以使用Python的GUI库Tkinter和一些第三方库来实现这个实时监控系统。以下是一个简单的代码示例:
```python
import tkinter as tk
from tkinter import ttk
import requests
import json
class WeatherApp:
def __init__(self, master):
self.master = master
master.title("实时天气监控系统")
self.location_label = tk.Label(master, text="输入城市:")
self.location_label.grid(row=0, column=0)
self.location_entry = tk.Entry(master)
self.location_entry.grid(row=0, column=1)
self.get_button = tk.Button(master, text="获取天气", command=self.get_weather)
self.get_button.grid(row=0, column=2)
self.temperature_label = tk.Label(master, text="温度:")
self.temperature_label.grid(row=1, column=0)
self.temperature_value = tk.Label(master, text="")
self.temperature_value.grid(row=1, column=1)
self.humidity_label = tk.Label(master, text="湿度:")
self.humidity_label.grid(row=2, column=0)
self.humidity_value = tk.Label(master, text="")
self.humidity_value.grid(row=2, column=1)
def get_weather(self):
location = self.location_entry.get()
url = f"http://api.openweathermap.org/data/2.5/weather?q={location}&appid=API_KEY"
response = requests.get(url)
data = json.loads(response.text)
temperature = round(data["main"]["temp"] - 273.15, 2)
humidity = data["main"]["humidity"]
self.temperature_value.config(text=str(temperature) + "℃")
self.humidity_value.config(text=str(humidity) + "%")
root = tk.Tk()
app = WeatherApp(root)
root.mainloop()
```
注意:上面的代码中的 API_KEY 部分需要替换为你自己的 OpenWeatherMap API 密钥。
这个代码会生成一个简单的GUI界面,你可以在里面输入城市名称并点击“获取天气”按钮来获取实时的温度和湿度数据。你可以根据自己的需要添加更多的功能和数据可视化方式。
相关推荐
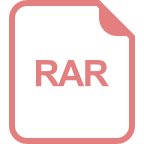
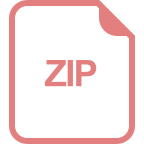
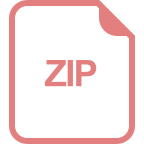
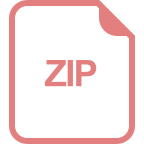
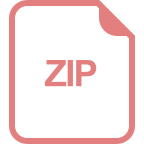
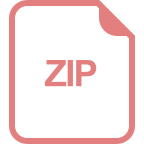
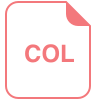
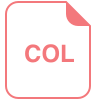
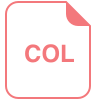
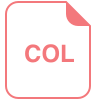
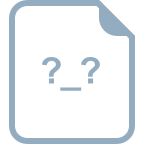
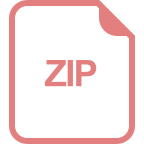
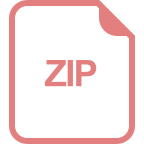
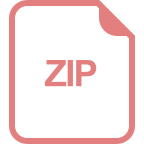
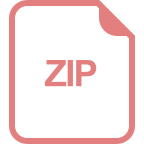