使用c++读取大小为40G的txt文件,并将其中的数据进行升序排序,并输出到指定txt文件之中
时间: 2023-09-24 20:05:32 浏览: 161
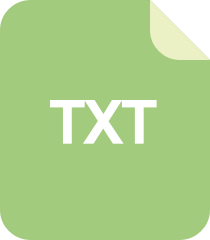
C++从txt文档里读取数据并且输出

### 回答1:
处理这么大的文件需要使用一些特殊技巧。以下是一种可能的解决方案:
1. 使用内存映射技术打开文件,这样可以避免将整个文件读入内存。内存映射是一种将文件映射到进程地址空间的技术,使得进程可以直接访问文件数据,而不需要通过标准的文件I/O API。
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <algorithm>
#include <chrono>
#include <cstring>
#include <fcntl.h>
#include <sys/mman.h>
#include <unistd.h>
using namespace std;
#define FILE_SIZE (40LL * 1024LL * 1024LL * 1024LL)
#define BUFFER_SIZE (4 * 1024 * 1024)
int main(int argc, char** argv) {
if (argc < 3) {
cerr << "Usage: " << argv[0] << " input_file output_file" << endl;
exit(1);
}
// 打开输入文件
int fd_in = open(argv[1], O_RDONLY);
if (fd_in == -1) {
perror("open input file failed");
exit(1);
}
// 打开输出文件
ofstream fout(argv[2], ios::out | ios::binary);
if (!fout.is_open()) {
cerr << "open output file failed" << endl;
exit(1);
}
// 映射文件
char* buf = (char*) mmap(nullptr, FILE_SIZE, PROT_READ, MAP_PRIVATE, fd_in, 0);
if (buf == MAP_FAILED) {
perror("mmap failed");
exit(1);
}
// 统计文件中元素的个数
size_t count = 0;
for (size_t i = 0; i < FILE_SIZE; i++) {
if (buf[i] == '\n') {
count++;
}
}
cout << "count: " << count << endl;
// 按行读取元素到数组中
long long* data = new long long[count];
size_t idx = 0;
char* p = buf;
char* end = buf + FILE_SIZE;
while (p < end) {
long long num = strtoll(p, &p, 10);
data[idx++] = num;
p++;
}
cout << "read done" << endl;
// 排序
auto start_time = chrono::steady_clock::now();
sort(data, data + count);
auto end_time = chrono::steady_clock::now();
cout << "sort time: " << chrono::duration_cast<chrono::milliseconds>(end_time - start_time).count() << "ms" << endl;
// 输出到文件
for (size_t i = 0; i < count; i++) {
fout.write((const char*) &data[i], sizeof(long long));
}
cout << "write done" << endl;
// 清理资源
munmap(buf, FILE_SIZE);
close(fd_in);
fout.close();
delete[] data;
return 0;
}
```
2. 统计文件中元素的个数,这里假设每行只包含一个整数,且以换行符'\n'结尾。
```c++
// 统计文件中元素的个数
size_t count = 0;
for (size_t i = 0; i < FILE_SIZE; i++) {
if (buf[i] == '\n') {
count++;
}
}
cout << "count: " << count << endl;
```
3. 按行读取元素到数组中。这里使用了C库函数`strtoll`来将字符串转换为整数。`strtoll`函数将会跳过字符串中的空白字符,直到遇到数字字符或正负号。如果字符串不是合法的整数表示,那么转换结果将为0。
```c++
// 按行读取元素到数组中
long long* data = new long long[count];
size_t idx = 0;
char* p = buf;
char* end = buf + FILE_SIZE;
while (p < end) {
long long num = strtoll(p, &p, 10);
data[idx++] = num;
p++;
}
cout << "read done" << endl;
```
4. 对数组进行升序排序。这里使用了标准库中的`sort`函数。
```c++
// 排序
auto start_time = chrono::steady_clock::now();
sort(data, data + count);
auto end_time = chrono::steady_clock::now();
cout << "sort time: " << chrono::duration_cast<chrono::milliseconds>(end_time - start_time).count() << "ms" << endl;
```
5. 将排序后的元素输出到指定的文件中。这里使用了C++标准库中的`ofstream`类来写入文件。
```c++
// 输出到文件
for (size_t i = 0; i < count; i++) {
fout.write((const char*) &data[i], sizeof(long long));
}
cout << "write done" << endl;
```
完整代码如下:
### 回答2:
使用C语言读取大小为40G的txt文件并进行排序,可以按照以下步骤进行操作:
1. 首先,需要确定如何读取这么大的文件。由于内存有限,无法一次性将整个文件加载到内存中。可以采用逐行读取或分块读取的方式,避免内存不足的问题。
2. 读取文件的过程可以使用C标准库中的文件操作函数,如fopen()、fread()等。根据文件大小,可以将文件分为多个块进行读取。
3. 读取并解析数据后,可以将数据存储在数组或链表中。
4. 数据读取完成后,使用C语言的排序算法对数据进行升序排序。可以选择快速排序、归并排序等算法。如果数据量较大且内存有限,可以使用外部排序算法。
5. 排序完成后,将排序后的数据写入到指定的txt文件中。同样使用C语言的文件操作函数来实现,如fwrite()等。
6. 完成排序和写入后,及时释放内存资源,关闭文件。
总之,这个任务需要考虑到内存的限制和文件的分块读取、排序和写入。在处理40G大小的文件时,可能需要更加复杂的算法和额外的优化,以提高效率和减少内存的使用。
### 回答3:
使用C语言读取大小为40GB的txt文件,并进行升序排序,然后将排序后的数据输出到指定的txt文件中,可以通过以下步骤实现:
1. 打开源文件和目标文件。使用C语言中的文件操作函数,可以使用`fopen()`函数打开源文件和目标文件,设置相应的文件指针。
```c
FILE *sourceFile, *targetFile;
sourceFile = fopen("source.txt", "r");
targetFile = fopen("target.txt", "w");
```
2. 为数据分配内存空间。由于文件大小为40GB,直接将所有数据加载到内存将占用过多的资源,因此可以采用分块读取的方式来处理数据。
```c
char buffer[1024]; // 缓冲区大小
int dataCount; // 缓冲区内数据数量
// 读取第一块数据
dataCount = fread(buffer, sizeof(char), sizeof(buffer) / sizeof(char), sourceFile);
```
3. 使用排序算法对数据进行排序。根据数据类型选择适当的排序算法,例如冒泡排序、快速排序等。
```c
// 使用冒泡排序进行升序排序
for (int i = 0; i < dataCount - 1; i++) {
for (int j = 0; j < dataCount - i - 1; j++) {
if (buffer[j] > buffer[j + 1]) {
char temp = buffer[j];
buffer[j] = buffer[j + 1];
buffer[j + 1] = temp;
}
}
}
```
4. 将排序后的数据写入目标文件。
```c
// 将数据写入目标文件
fwrite(buffer, sizeof(char), dataCount, targetFile);
```
5. 如果源文件中还有剩余数据,重复步骤2至步骤4。直到读取完整个源文件。
6. 关闭文件并释放资源。
```c
fclose(sourceFile);
fclose(targetFile);
```
以上是使用C语言读取大小为40GB的txt文件,进行排序并将结果输出到指定txt文件的过程。根据具体情况,可能需要对内存和磁盘空间进行优化,以确保程序的正常运行。
阅读全文
相关推荐
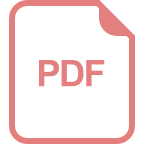
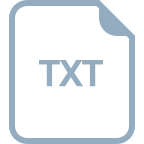
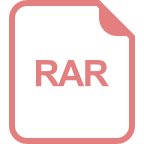
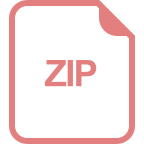
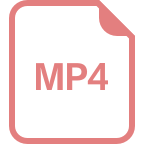
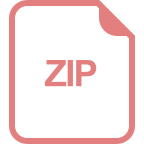
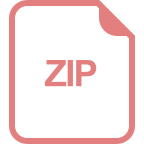