html+css+js 动漫留言板
时间: 2023-08-28 11:06:47 浏览: 93
以下是一个简单的 HTML+CSS+JS 动漫留言板的示例:
HTML代码:
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>动漫留言板</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="wrapper">
<h1>动漫留言板</h1>
<form>
<input type="text" name="name" placeholder="昵称">
<textarea name="message" placeholder="留言"></textarea>
<button type="submit">提交</button>
</form>
<ul class="messages">
<!-- 留言列表 -->
</ul>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS代码:
```
body {
font-family: "Microsoft YaHei", sans-serif;
background-color: #f5f5f5;
}
.wrapper {
max-width: 600px;
margin: 0 auto;
padding: 30px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
margin: 0 0 20px;
}
form input,
form textarea {
display: block;
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: none;
border-radius: 5px;
box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
}
form textarea {
height: 100px;
resize: none;
}
form button {
display: block;
margin: 0 auto;
padding: 10px 20px;
border: none;
border-radius: 5px;
background-color: #428bca;
color: #fff;
cursor: pointer;
}
.messages {
margin-top: 30px;
padding: 0;
list-style: none;
}
.messages li {
padding: 20px;
margin-bottom: 10px;
background-color: #f5f5f5;
box-shadow: 0 0 5px rgba(0, 0, 0, 0.1);
}
.messages li .name {
font-weight: bold;
}
.messages li .time {
font-size: 12px;
color: #999;
}
```
JS代码:
```
// 获取元素
const form = document.querySelector('form');
const messages = document.querySelector('.messages');
// 提交表单时执行
form.addEventListener('submit', function(e) {
e.preventDefault(); // 阻止默认提交行为
// 获取表单数据
const name = this.elements.name.value.trim();
const message = this.elements.message.value.trim();
// 检查是否为空
if (!name || !message) {
alert('昵称和留言不能为空!');
return;
}
// 构造留言结构
const li = document.createElement('li');
li.innerHTML = '<span class="name">' + name + '</span><span class="time">' + getTime() + '</span><p>' + message + '</p>';
// 添加到留言列表
messages.appendChild(li);
// 清空表单
this.reset();
});
// 获取当前时间
function getTime() {
const now = new Date();
const year = now.getFullYear();
const month = now.getMonth() + 1;
const date = now.getDate();
const hour = now.getHours();
const minute = now.getMinutes();
const second = now.getSeconds();
return year + '-' + padZero(month) + '-' + padZero(date) + ' ' + padZero(hour) + ':' + padZero(minute) + ':' + padZero(second);
}
// 补零
function padZero(num) {
return num < 10 ? '0' + num : num;
}
```
该留言板使用了 HTML 表单、CSS 样式和 JavaScript 脚本,能够实现用户提交留言并显示在留言列表中,同时也会显示留言的作者和时间。你可以根据自己的需要进行修改和完善。
相关推荐
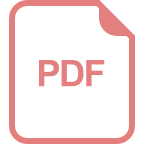














