C#如何使用同一个CSV文件循环读取每一行,全部找到并清空带有指定字符串的那一行数据
时间: 2023-09-10 10:11:28 浏览: 50
你可以使用C#中的`StreamReader`和`StreamWriter`类来读写CSV文件,并使用`string`类的`Contains`方法检查每一行是否包含指定字符串。以下是一个示例代码,可以循环读取CSV文件中的每一行,如果某一行包含指定字符串则清空该行数据:
```csharp
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
string filePath = "path/to/your/csv/file.csv";
string searchStr = "your search string";
// create a temporary file to store modified data
string tempFilePath = Path.GetTempFileName();
try
{
using (var reader = new StreamReader(filePath))
{
using (var writer = new StreamWriter(tempFilePath))
{
// read and process each line of the CSV file
while (!reader.EndOfStream)
{
string line = reader.ReadLine();
// check if the line contains the search string
if (line.Contains(searchStr))
{
// clear the line by writing an empty string to the temporary file
writer.WriteLine("");
}
else
{
// write the original line to the temporary file
writer.WriteLine(line);
}
}
}
}
// replace the original CSV file with the modified data
File.Delete(filePath);
File.Move(tempFilePath, filePath);
}
catch (Exception ex)
{
Console.WriteLine("Error: " + ex.Message);
}
}
}
```
请注意,此代码将修改原始CSV文件。因此,您应该在处理之前备份文件,以便在出现问题时可以还原数据。
相关推荐
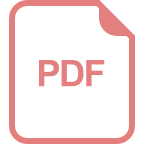
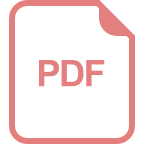














