c# 查找数据库一张表里的等于5的数字并把这一行储存在列表里
时间: 2024-03-27 11:36:07 浏览: 57
好的,可以使用以下代码实现:
``` csharp
using System.Data.SqlClient;
using System.Collections.Generic;
// 定义一个存储结果的类
public class Result
{
public int Column1 { get; set; }
public string Column2 { get; set; }
// 其他列...
}
// 连接数据库
string connectionString = "Data Source=myServerAddress;Initial Catalog=myDataBase;User ID=myUsername;Password=myPassword;";
SqlConnection connection = new SqlConnection(connectionString);
connection.Open();
// 查询数据
string commandText = "SELECT * FROM MyTable WHERE Column1 = 5;";
SqlCommand command = new SqlCommand(commandText, connection);
SqlDataReader reader = command.ExecuteReader();
// 将结果存入列表
List<Result> results = new List<Result>();
while (reader.Read())
{
Result result = new Result();
result.Column1 = reader.GetInt32(0);
result.Column2 = reader.GetString(1);
// 其他列...
results.Add(result);
}
// 关闭连接
reader.Close();
connection.Close();
```
其中,`MyTable` 是要查询的表名,`Column1`是等于5的数字所在的列名,`Column2`是需要存储的其他列名,可以根据需要修改。注意,要替换掉连接字符串中的服务器地址、数据库名、用户名和密码,以及修改 `Result` 类的属性和构造函数中的列索引。
阅读全文
相关推荐
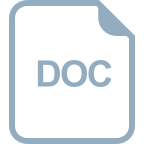
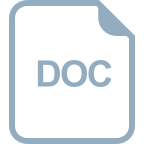
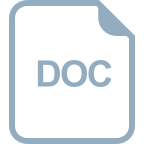
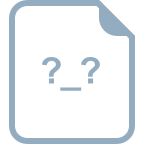
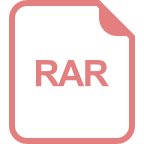
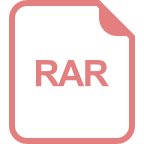
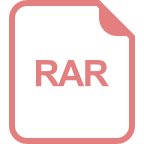
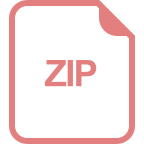
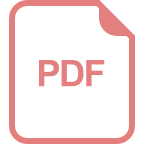
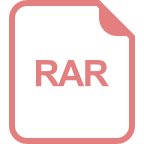
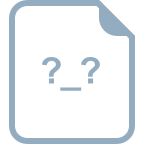
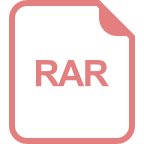
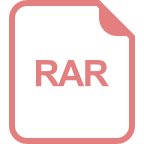
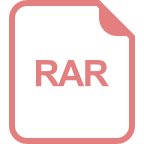
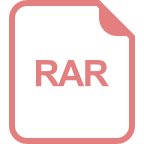
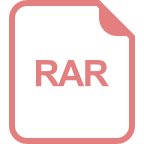
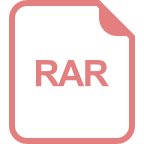