利用键盘回调函数实现二维图形的几何变换(旋转、平移、缩放) 变换的对象自选,实现8个键盘响应(退出、还原、旋转2、平移2、缩放2) 注:请在程序运行的黑色文本框里,给出操作说明
时间: 2023-07-10 13:14:08 浏览: 183
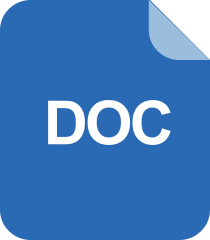
图形变换行旋转、缩放、平移变换。

好的,以下是基于键盘回调函数实现二维图形几何变换的示例代码,其中变换对象为一个矩形:
```c++
#include <stdio.h>
#include <math.h>
#include <windows.h>
#include <GL/glut.h>
// 矩形的四个顶点坐标
GLfloat vertices[][2] = {{-1.0, -1.0}, {1.0, -1.0}, {1.0, 1.0}, {-1.0, 1.0}};
GLfloat theta = 0.0; // 旋转角度
GLfloat tx = 0.0, ty = 0.0; // 平移距离
GLfloat sx = 1.0, sy = 1.0; // 缩放比例
// 绘制矩形
void drawRect() {
glClear(GL_COLOR_BUFFER_BIT);
glColor3f(1.0, 1.0, 1.0);
glBegin(GL_POLYGON);
for (int i = 0; i < 4; i++) {
glVertex2fv(vertices[i]);
}
glEnd();
glFlush();
}
// 初始化OpenGL
void init() {
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(-2.0, 2.0, -2.0, 2.0);
glMatrixMode(GL_MODELVIEW);
}
// 旋转函数
void rotate() {
GLfloat tempX, tempY;
for (int i = 0; i < 4; i++) {
tempX = vertices[i][0] * cos(theta) - vertices[i][1] * sin(theta);
tempY = vertices[i][0] * sin(theta) + vertices[i][1] * cos(theta);
vertices[i][0] = tempX;
vertices[i][1] = tempY;
}
glutPostRedisplay();
}
// 平移函数
void translate() {
for (int i = 0; i < 4; i++) {
vertices[i][0] += tx;
vertices[i][1] += ty;
}
glutPostRedisplay();
}
// 缩放函数
void scale() {
for (int i = 0; i < 4; i++) {
vertices[i][0] *= sx;
vertices[i][1] *= sy;
}
glutPostRedisplay();
}
// 键盘回调函数
void keyboard(unsigned char key, int x, int y) {
switch (key) {
case 'q': // 退出
exit(0);
break;
case 'r': // 还原
theta = 0.0;
tx = ty = 0.0;
sx = sy = 1.0;
for (int i = 0; i < 4; i++) {
vertices[i][0] = -1.0 + i / 2.0;
vertices[i][1] = -1.0 + i % 2 * 2.0;
}
glutPostRedisplay();
break;
case '2': // 旋转2
theta += 0.1;
rotate();
break;
case '4': // 平移2
tx -= 0.1;
translate();
break;
case '6': // 平移2
tx += 0.1;
translate();
break;
case '8': // 缩放2
sx *= 1.1;
sy *= 1.1;
scale();
break;
case '5': // 缩放2
sx /= 1.1;
sy /= 1.1;
scale();
break;
}
}
// 主函数
int main(int argc, char **argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE | GLUT_RGB);
glutInitWindowSize(500, 500);
glutCreateWindow("2D Transformation");
init();
glutDisplayFunc(drawRect);
glutKeyboardFunc(keyboard);
glutMainLoop();
return 0;
}
```
操作说明:
- q:退出程序
- r:还原矩形的位置、旋转角度和缩放比例
- 2:将矩形逆时针旋转0.1弧度
- 4:将矩形左移0.1个单位
- 6:将矩形右移0.1个单位
- 8:将矩形按中心点放大1.1倍
- 5:将矩形按中心点缩小1.1倍
以上操作均会在黑色窗口中实时显示出来。
阅读全文
相关推荐
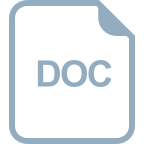
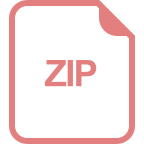
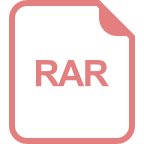
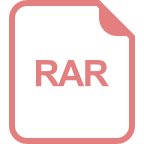
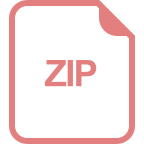
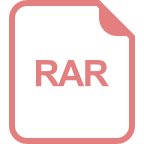
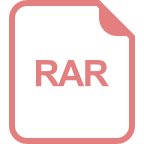
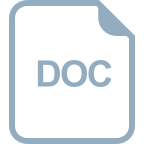
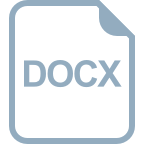
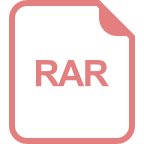
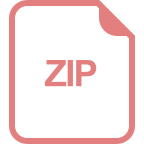
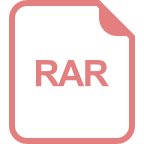
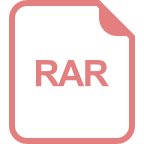
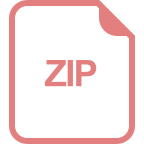
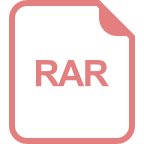
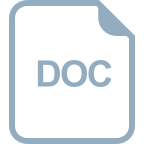
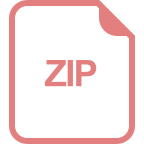